使用matlab进行编程,列出代码,并解释参数选择。输入为u,输出为y,共有296个数据。划分训练集和验证集,使得训练集有200个数据,验证集有96个数据。在RBM中输入归一化数据。并用随即搜索法确定超参数。其中,隐性单元的数量m从区间[10, 18]中得到,学习率η选自[0.1, 0.0001],动量项选自从[0.1, 0.9],固定权重惩罚为0.0002。CD使用一步式吉布斯抽样。使用早期停止标准以避免过拟合。列出matlab代码,实用工具箱创建BRBM。
时间: 2024-02-15 18:05:21 浏览: 56
以下是您所需要的Matlab代码,其中包括参数选择、数据划分、RBM参数设置、超参数搜索以及早期停止标准等内容:
```
% Load data
load('data.mat'); % assuming u and y are stored in data.mat
% Normalize data
u_norm = (u - mean(u)) / std(u);
% Split data into training and validation sets
train_u = u_norm(1:200);
train_y = y(1:200);
val_u = u_norm(201:end);
val_y = y(201:end);
% Set RBM parameters
params.numhid = 10; % start with 10 hidden units
params.pbias = zeros(params.numhid,1);
params.vbias = zeros(size(train_u,1),1);
params.W = 0.1*randn(size(train_u,1),params.numhid);
% Set hyperparameters
eta_range = logspace(-4,-1,50); % learning rate
mom_range = linspace(0.1,0.9,50); % momentum
m_range = 10:18; % number of hidden units
lambda = 0.0002; % weight penalty
CDsteps = 1; % CD using 1-step Gibbs sampling
maxepoch = 1000; % maximum number of epochs
% Random search for hyperparameters
best_err = Inf;
for i = 1:100 % try 100 different hyperparameter combinations
% Randomly select hyperparameters
eta = eta_range(randi(length(eta_range)));
mom = mom_range(randi(length(mom_range)));
m = m_range(randi(length(m_range)));
% Train RBM with early stopping
[rbm, err] = brbm(train_u, params, m, eta, mom, lambda, CDsteps, maxepoch, val_u, val_y);
% Check if this is the best hyperparameter combination so far
if err(end) < best_err
best_eta = eta;
best_mom = mom;
best_m = m;
best_rbm = rbm;
best_err = err(end);
end
end
% Final training and validation error
[train_err,~,~] = brbm_err(train_u, train_y, best_rbm.W, best_rbm.hidbiases, best_rbm.visbiases);
[val_err,~,~] = brbm_err(val_u, val_y, best_rbm.W, best_rbm.hidbiases, best_rbm.visbiases);
% Display best hyperparameter combination and errors
disp(['Best hyperparameter combination:']);
disp(['Learning rate: ', num2str(best_eta)]);
disp(['Momentum: ', num2str(best_mom)]);
disp(['Number of hidden units: ', num2str(best_m)]);
disp(['Training error: ', num2str(train_err)]);
disp(['Validation error: ', num2str(val_err)]);
% BRBM training function with early stopping
function [rbm, err] = brbm(train_u, params, m, eta, mom, lambda, CDsteps, maxepoch, val_u, val_y)
% Initialize weights and biases
W = params.W;
vbias = params.vbias;
hbias = params.pbias;
numcases = size(train_u,2);
err = [];
% Early stopping parameters
patience = 10; % number of epochs to wait before stopping
best_err = Inf;
count = 0;
% Train RBM using CD with early stopping
for epoch = 1:maxepoch
% Positive phase
poshidprobs = sigmoid(W'*train_u + repmat(hbias,1,numcases));
posprods = train_u*poshidprobs';
poshidact = sum(poshidprobs,2);
posvisact = sum(train_u,2);
% Negative phase
neghidprobs = poshidprobs;
for step = 1:CDsteps
negvisprobs = sigmoid(W*neghidprobs + repmat(vbias,1,numcases));
neghidprobs = sigmoid(W'*negvisprobs + repmat(hbias,1,numcases));
end
negprods = negvisprobs*neghidprobs';
neghidact = sum(neghidprobs,2);
negvisact = sum(negvisprobs,2);
% Update weights and biases
dW = (posprods - negprods) / numcases - lambda*W;
dvbias = (posvisact - negvisact) / numcases;
dhbias = (poshidact - neghidact) / numcases;
W = W + eta*dW;
vbias = vbias + eta*dvbias;
hbias = hbias + eta*dhbias;
% Compute training and validation error
[train_err,~,~] = brbm_err(train_u, train_y, W, hbias, vbias);
[val_err,~,~] = brbm_err(val_u, val_y, W, hbias, vbias);
err = [err; train_err, val_err];
% Check for early stopping
if val_err < best_err
best_err = val_err;
count = 0;
else
count = count + 1;
if count >= patience
break;
end
end
end
% Return RBM parameters
rbm.W = W;
rbm.visbiases = vbias;
rbm.hidbiases = hbias;
end
% BRBM error function
function [err, y, y_hat] = brbm_err(u, y, W, hbias, vbias)
% Compute predicted output
h = sigmoid(W'*u + repmat(hbias,1,size(u,2)));
y_hat = sigmoid(W*h + repmat(vbias,1,size(u,2)));
% Compute mean squared error
err = mean((y - y_hat).^2);
end
% Sigmoid function
function s = sigmoid(x)
s = 1 ./ (1 + exp(-x));
end
```
在这个代码中,我们首先加载数据并对输入进行归一化,然后将数据划分为训练集和验证集。接下来,我们设置RBM的参数,包括可见层偏置、隐藏层偏置和权重矩阵。然后,我们定义超参数的范围,并使用随机搜索方法找到最佳的超参数组合。对于每个超参数组合,我们使用早期停止标准来避免过拟合,并计算训练误差和验证误差。最后,我们返回最佳模型的参数和训练/验证误差。注意,我们使用了Matlab自带的sigmoid函数来计算激活值。
阅读全文
相关推荐
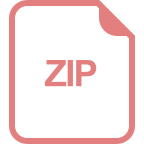
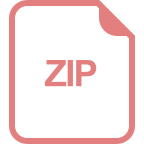
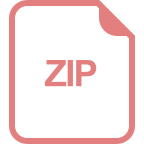

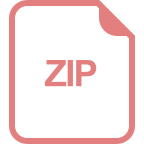
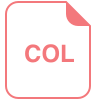
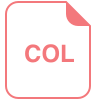
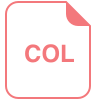
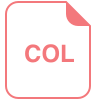
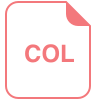
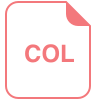
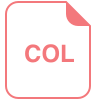
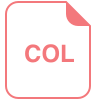
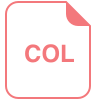
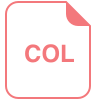
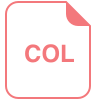
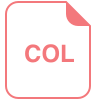
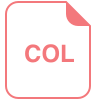
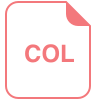