ValueError: Found array with dim 4. DBSCAN expected <= 2.
时间: 2024-03-03 20:50:18 浏览: 113
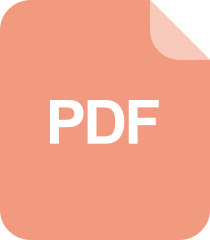
python3.7解决最小二乘遇到ValueError:Expected 2D array, got 1D array instead: array=[5.].关于reshape和predict
这个错误是因为DBSCAN算法默认只能处理二维数据(即特征数量不超过2),但是我们使用ResNet50模型提取的特征向量是四维的。因此,需要对特征向量进行降维处理。
可以使用主成分分析(PCA)或t-SNE等降维方法将特征向量降维为二维,然后再使用DBSCAN进行聚类。下面是修改后的代码:
```
import os
import numpy as np
from PIL import Image
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input
from sklearn.cluster import DBSCAN
from sklearn.decomposition import PCA
# 加载 ResNet50 模型
model = ResNet50(weights='imagenet', include_top=False)
# 加载并预处理图片,提取特征向量
features = []
for filename in os.listdir('D:/wjd/2'):
if filename.endswith('.png'):
img = Image.open(os.path.join('D:/wjd/2', filename))
img = img.convert('RGB') # 将 RGBA 转换为 RGB
img = img.resize((224, 224))
x = np.array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
feature = model.predict(x)
feature = np.squeeze(feature)
features.append(feature)
# 将特征向量进行降维处理
pca = PCA(n_components=2)
features_pca = pca.fit_transform(features)
# 将特征向量进行聚类,自动确定聚类的数量
dbscan = DBSCAN(eps=0.5, min_samples=2).fit(features_pca)
n_clusters = len(set(dbscan.labels_))
# 将每张图片分配到对应的聚类中
for i, label in enumerate(dbscan.labels_):
filename = os.listdir('D:/wjd/2')[i]
print('{} belongs to cluster {}'.format(filename, label))
```
在这个版本的代码中,我们使用PCA将特征向量降维为二维,然后再使用DBSCAN进行聚类。
阅读全文
相关推荐
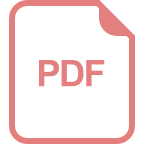
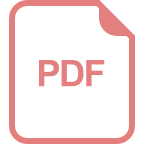














