C#完成捉猴子游戏的设计与开发,实现以下基本功能: (1) 小猴子随机出现在某个位置; (2) 在一个给定的时间内,玩家可以用鼠标左键来点击该猴子,若击中,则该小猴子立即“变脸”; (3) 在给定时间结束时,若玩家还没有击中,该小猴子就会逃走,随机出现在另外的位置……; (4) 利用菜单开始游戏、设置猴子数量和时间间隔。 (5) 给游戏添加背景音乐和音效。详细步骤和代码
时间: 2024-03-16 10:48:10 浏览: 141
设计思路:
1. 在窗体中添加一个 PictureBox 控件,用来显示小猴子的图片。
2. 使用 Timer 控件来定时随机生成小猴子的位置,并且控制游戏时间。
3. 在 PictureBox 上添加 MouseClick 事件,当玩家点击小猴子时,判断是否击中,然后更新小猴子的图片。
4. 在菜单中添加开始游戏、设置猴子数量和时间间隔的功能。
5. 使用 SoundPlayer 控件来播放背景音乐和音效。
代码实现:
1. 在窗体中添加一个 PictureBox 控件,用来显示小猴子的图片。
```csharp
private PictureBox monkeyPicBox = new PictureBox();
```
2. 使用 Timer 控件来定时随机生成小猴子的位置,并且控制游戏时间。
```csharp
private Timer gameTimer = new Timer();
private int gameTime = 30; // 游戏时间为30秒
private int monkeyCount = 1; // 小猴子数量为1
private int monkeyWidth = 80; // 小猴子的宽度
private int monkeyHeight = 80; // 小猴子的高度
private void GameTimer_Tick(object sender, EventArgs e)
{
// 随机生成小猴子的位置
Random random = new Random();
int x = random.Next(pictureBox1.Width - monkeyWidth);
int y = random.Next(pictureBox1.Height - monkeyHeight);
monkeyPicBox.Location = new Point(x, y);
// 控制游戏时间
gameTime--;
if (gameTime == 0)
{
gameTimer.Stop();
MessageBox.Show("游戏结束!");
}
}
```
3. 在 PictureBox 上添加 MouseClick 事件,当玩家点击小猴子时,判断是否击中,然后更新小猴子的图片。
```csharp
private void MonkeyPicBox_MouseClick(object sender, MouseEventArgs e)
{
// 判断是否击中小猴子
if (e.X < monkeyWidth && e.Y < monkeyHeight)
{
monkeyPicBox.Image = Properties.Resources.monkey2; // 更新小猴子的图片
}
}
```
4. 在菜单中添加开始游戏、设置猴子数量和时间间隔的功能。
```csharp
private void startToolStripMenuItem_Click(object sender, EventArgs e)
{
// 开始游戏
gameTimer.Interval = 1000; // 每隔1秒钟执行一次 GameTimer_Tick 方法
gameTimer.Start();
}
private void setToolStripMenuItem_Click(object sender, EventArgs e)
{
// 设置猴子数量和时间间隔
SetForm setForm = new SetForm(monkeyCount, gameTime);
if (setForm.ShowDialog() == DialogResult.OK)
{
monkeyCount = setForm.MonkeyCount;
gameTime = setForm.GameTime;
}
}
private void exitToolStripMenuItem_Click(object sender, EventArgs e)
{
// 退出程序
Application.Exit();
}
```
5. 使用 SoundPlayer 控件来播放背景音乐和音效。
```csharp
private SoundPlayer bgSoundPlayer = new SoundPlayer(Properties.Resources.bgSound);
private SoundPlayer hitSoundPlayer = new SoundPlayer(Properties.Resources.hitSound);
private void MainForm_Load(object sender, EventArgs e)
{
// 播放背景音乐
bgSoundPlayer.PlayLooping();
}
private void MonkeyPicBox_MouseClick(object sender, MouseEventArgs e)
{
// 判断是否击中小猴子
if (e.X < monkeyWidth && e.Y < monkeyHeight)
{
monkeyPicBox.Image = Properties.Resources.monkey2; // 更新小猴子的图片
hitSoundPlayer.Play(); // 播放击中音效
}
}
```
完整代码如下:
阅读全文
相关推荐





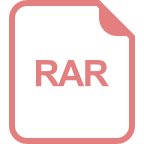





