BeanUtils.copyProperties浅拷贝代码示例
时间: 2023-10-12 17:20:44 浏览: 187
假设有以下两个类:
```java
public class User {
private String name;
private int age;
// getter and setter methods
}
public class UserVO {
private String name;
private int age;
// getter and setter methods
}
```
使用 `BeanUtils.copyProperties()` 方法可以将 `User` 类的实例对象的属性值拷贝到 `UserVO` 类的实例对象中。
```java
User user = new User();
user.setName("张三");
user.setAge(20);
UserVO userVO = new UserVO();
BeanUtils.copyProperties(user, userVO);
```
这样,`userVO` 对象就拥有了 `user` 对象的属性值。需要注意的是,`BeanUtils.copyProperties()` 方法进行的是浅拷贝,即如果属性值是对象类型,只会拷贝引用,而不是对象本身。
相关问题
beanutils.copyproperties是浅拷贝吗
是的,BeanUtils.copyProperties方法是浅拷贝。浅拷贝只是将对象的引用复制给了另一个对象,而不是复制对象本身。因此,如果原始对象中的属性是引用类型,则新对象和原始对象将共享相同的引用,这可能会导致意外的行为。以下是一个示例,演示了BeanUtils.copyProperties方法的浅拷贝行为:
```java
public class Person {
private String name;
private Address address;
// getters and setters
}
public class Address {
private String city;
private String state;
// getters and setters
}
// 测试代码
Person person1 = new Person();
person1.setName("John");
Address address1 = new Address();
address1.setCity("New York");
address1.setState("NY");
person1.setAddress(address1);
Person person2 = new Person();
BeanUtils.copyProperties(person2, person1);
// 修改person1的地址信息
person1.getAddress().setCity("Los Angeles");
// person2的地址信息也被修改了,因为它们共享相同的Address对象
System.out.println(person2.getAddress().getCity()); // 输出:Los Angeles
```
beanutils.copyproperties是深拷贝还是浅拷贝
`BeanUtils.copyProperties`方法是浅拷贝,而不是深拷贝。
浅拷贝意味着它会将源对象的属性值复制到目标对象中,但如果属性值是引用类型对象,那么目标对象和源对象将引用同一个对象。这意味着如果修改一个对象的属性值,另一个对象的属性值也会被修改。
如果你需要进行深拷贝,即复制对象及其引用类型属性的所有层级,你可以考虑使用其他方法或工具来实现。一种常见的做法是通过序列化和反序列化来实现深拷贝,或者使用第三方库如Apache Commons Lang的`SerializationUtils.clone`方法。
以下是使用Apache Commons Lang库实现深拷贝的示例代码:
```java
import org.apache.commons.lang3.SerializationUtils;
public class DeepCopyExample {
public static void main(String[] args) {
SourceObject source = new SourceObject();
source.setName("John");
source.setAge(25);
DestinationObject destination = SerializationUtils.clone(source);
System.out.println("Source: " + source.getName() + ", " + source.getAge());
System.out.println("Destination: " + destination.getName() + ", " + destination.getAge());
// 修改源对象的属性值
source.setName("Alice");
source.setAge(30);
System.out.println("Source: " + source.getName() + ", " + source.getAge());
System.out.println("Destination: " + destination.getName() + ", " + destination.getAge());
}
}
class SourceObject implements Serializable {
private String name;
private int age;
// 省略 getter 和 setter 方法
}
class DestinationObject implements Serializable {
private String name;
private int age;
// 省略 getter 和 setter 方法
}
```
这个示例中,通过使用`SerializationUtils.clone`方法对源对象进行深拷贝,确保了目标对象与源对象是独立的。修改源对象的属性值不会影响到目标对象。
希望这能解答你的问题!如果还有任何疑问,请随时提问。
阅读全文
相关推荐




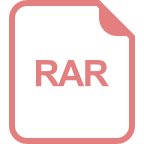

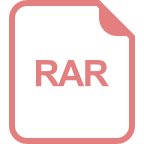
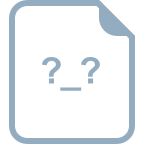
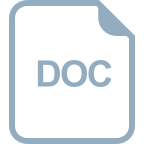
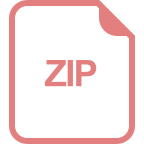
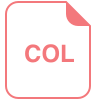
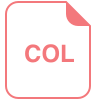
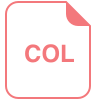
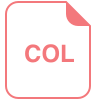