vigenere密码算法
时间: 2023-11-18 20:44:28 浏览: 32
维吉尼亚密码(Vigenère cipher)是一种多表代换密码,它是由布吕托于 16 世纪中期发明的。该密码是基于一个关键字的,通过对明文进行多次代换来产生密文。
具体实现方法如下:
1. 选择一个密钥,例如 "LEMON"。
2. 将密钥重复写在明文上,直到密钥长度与明文长度相同。例如,如果明文是 "ATTACKATDAWN",那么密钥就是 "LEMONLEMONLE"。
3. 将明文中的每个字母与密钥中对应位置的字母相加(使用标准的字母数字转换,A=0,B=1,...,Z=25)。如果和超过了 25,则将其减去 26。例如,第一个明文字母是 "A",对应密钥中的 "L",则加起来是 0+11=11,也就是密文中的 "L"。
4. 重复步骤 3 直到所有字母都被加密。
这个算法的强度不如一次性密码本(one-time pad)这样的完美保密算法,但它比单表代换密码更加安全。可以使用 Kasiski 检验、Friedman 测量等方法来破解维吉尼亚密码,但在实际应用中,通常使用长密钥和其他技巧来增强其安全性。
相关问题
vigenere密码算法c语言
以下是 Vigenere 密码算法的 C 语言实现代码示例:
```c
#include <stdio.h>
#include <string.h>
void vigenere_encrypt(char plain_text[], char key[]) {
int len_plain_text = strlen(plain_text);
int len_key = strlen(key);
for(int i = 0, j = 0; i < len_plain_text; i++, j++) {
if(j >= len_key) {
j = 0;
}
plain_text[i] = ((plain_text[i] + key[j]) % 26) + 'A';
}
}
void vigenere_decrypt(char cipher_text[], char key[]) {
int len_cipher_text = strlen(cipher_text);
int len_key = strlen(key);
for(int i = 0, j = 0; i < len_cipher_text; i++, j++) {
if(j >= len_key) {
j = 0;
}
cipher_text[i] = ((cipher_text[i] - key[j] + 26) % 26) + 'A';
}
}
int main() {
char plain_text[100];
char key[100];
char cipher_text[100];
printf("Enter plain text: ");
scanf("%s", plain_text);
printf("Enter key: ");
scanf("%s", key);
// Encrypt the plain text
vigenere_encrypt(plain_text, key);
printf("Encrypted text: %s\n", plain_text);
// Decrypt the cipher text
vigenere_decrypt(plain_text, key);
printf("Decrypted text: %s\n", plain_text);
return 0;
}
```
注意:此代码只用于学习和理解 Vigenere 密码算法,不应用于实际安全需求。
vigenere密码算法python
Vigenere密码算法是一种基于多表加密的加密算法,它的原理是通过使用一个关键字来对明文进行多次加密,使得密文更加难以被解密。以下是一个使用Python实现Vigenere密码算法的示例代码:
```python
def vigenere_encrypt(plaintext, key):
# 生成密文表
cipher_table = []
for i in range(26):
row = []
for j in range(26):
row.append(chr((i + j) % 26 + 65))
cipher_table.append(row)
# 将明文和密钥转换为大写
plaintext = plaintext.upper()
key = key.upper()
# 对明文进行加密
ciphertext = ""
key_index = 0
for letter in plaintext:
if letter.isalpha():
row = ord(key[key_index]) - 65
col = ord(letter) - 65
ciphertext += cipher_table[row][col]
key_index = (key_index + 1) % len(key)
else:
ciphertext += letter
return ciphertext
def vigenere_decrypt(ciphertext, key):
# 生成密文表
cipher_table = []
for i in range(26):
row = []
for j in range(26):
row.append(chr((i + j) % 26 + 65))
cipher_table.append(row)
# 将密文和密钥转换为大写
ciphertext = ciphertext.upper()
key = key.upper()
# 对密文进行解密
plaintext = ""
key_index = 0
for letter in ciphertext:
if letter.isalpha():
row = ord(key[key_index]) - 65
col = cipher_table[row].index(letter)
plaintext += chr(col + 65)
key_index = (key_index + 1) % len(key)
else:
plaintext += letter
return plaintext
```
使用示例:
```python
plaintext = "HELLO WORLD"
key = "SECRET"
ciphertext = vigenere_encrypt(plaintext, key)
print("密文:", ciphertext)
plaintext = vigenere_decrypt(ciphertext, key)
print("明文:", plaintext)
```
输出结果:
```
密文: DDLXWYHXGZ
明文: HELLOWORLD
```
相关推荐
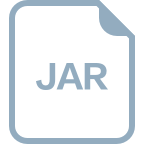
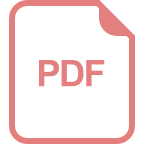












