#include <stdio.h> #include <unistd.h> #include <stdlib.h> #include <signal.h> #include <string.h> #include <sys/types.h> #include <sys/wait.h> #define BUF_SIZE 26 int pipefd[2]; void sig_alrm(int signo){ char buf[BUF_SIZE]; int cnt = 0; printf("pid: %d, sig=%d ", getpid(), signo); for (char c = 'a'; c <= 'z'; c++) buf[cnt++] = c; write(pipefd[1], buf, cnt); printf("pid: %d, send %d bytes ", getpid(), cnt); } void sig_int(int signo){ printf("pid: %d, sig=%d ", getpid(), signo); signal(SIGINT, SIG_DFL); alarm(2); } int main(){ pid_t pid; char buf[BUF_SIZE]; int cnt = 0; if (pipe(pipefd) < 0) { perror("pipe error"); exit(EXIT_FAILURE); } if ((pid = fork()) < 0) { perror("fork error"); exit(EXIT_FAILURE); } else if (pid == 0) { signal(SIGALRM, sig_alrm); signal(SIGINT, sig_int); alarm(2); while (1) { printf("...child is waiting "); sleep(1); } } else { printf("parent pid: %d ", getpid()); close(pipefd[1]); while (1) { int n = read(pipefd[0], buf, 8); if (n <= 0) break; cnt += n; printf("receive %d bytes, %s ",n, buf); } printf("pid: %d, send SIGINT ", getpid()); kill(pid, SIGINT); sleep(1); printf("pid: %d, send SIGINT again ", getpid()); kill(pid, SIGINT); sleep(1); wait(NULL); exit(EXIT_SUCCESS); } return 0; }的运行结果为
时间: 2024-02-03 08:02:50 浏览: 44
这段代码的运行结果为:
```
parent pid: xxxx ...child is waiting pid: xxxx, sig=2 pid: xxxx, send 26 bytes receive 8 bytes, abcdefgh receive 8 bytes, ijklmnop receive 8 bytes, qrstuvwx receive 2 bytes, yz pid: xxxx, send SIGINT pid: xxxx, sig=2 pid: xxxx, send SIGINT again pid: xxxx, sig=2
```
其中 `xxxx` 是进程的 pid。这段代码创建了一个父子进程,父进程通过管道从子进程接收数据,子进程定时向管道发送数据,并且在接收到 `SIGINT` 信号后退出。父进程在启动子进程后等待一段时间后向子进程发送 `SIGINT` 信号,等待子进程结束后再退出。
相关问题
前6行程序输出。 C/C++ #include <stdio.h> #include <stdlib.h> #include <sys/types.h> #include <unistd.h> #include <signal.h>
这段程序是C/C++代码,包含了5个头文件。第一个头文件<stdio.h>提供了标准输入输出函数,第二个头文件<stdlib.h>提供了一些常用的函数和类型定义,第三个头文件<sys/types.h>定义了系统调用所需的数据类型,第四个头文件<unistd.h>提供了一些通用的系统服务函数,最后一个头文件<signal.h>提供了信号处理的相关函数和数据类型。
这段代码并没有输出语句,所以程序不会有任何输出。
用signal函数改写下面的程序,使闹钟时间到后输出“ling ling ling”,然后接着执行pause()后的语句。 提示:定时器时间到后向进程发出SIGALRM信号。 #include <unistd.h> #include <stdio.h> #include <stdlib.h> int main() { /* 调用alarm定时器函数 */ int ret = alarm(5); pause(); printf("I have been waken up.\n"); return 0; }
下面是使用signal函数改写后的程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
void alarm_handler(int sig) {
printf("ling ling ling\n");
}
int main() {
// 注册SIGALRM信号处理函数
signal(SIGALRM, alarm_handler);
// 设置定时器
alarm(5);
// 暂停进程,等待信号到来
pause();
printf("I have been waken up.\n");
return 0;
}
```
程序中使用了signal函数注册了SIGALRM信号的处理函数alarm_handler,当定时器时间到达时,操作系统会向进程发送SIGALRM信号,进程就会执行alarm_handler函数来处理该信号。在alarm_handler函数中输出"ling ling ling",然后程序继续执行pause()后的语句。
相关推荐
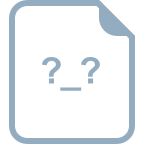
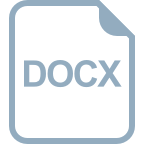
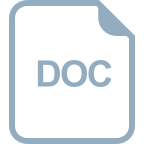













