遍历文件夹下面的所有xlsx文件,遍历每个xlsx文件中的工作表,遍历工作表中的每一个单元格,查找单元格中含有"张三"的文件,并打印出名字。并把文件复制到aa文件夹下。
时间: 2023-09-04 19:05:21 浏览: 181
### 回答1:
为了遍历文件夹下的所有xlsx文件,我们可以使用Python的os模块和glob模块。首先,我们可以使用os模块的listdir()函数列出文件夹下的所有文件,然后使用glob模块匹配所有的.xlsx文件。
接下来,我们可以使用openpyxl模块来读取每个xlsx文件,并遍历其中的每个工作表。对于每个工作表,我们可以使用循环来遍历其中的每个单元格,检查它们是否包含指定的字符串。如果找到了包含该字符串的单元格,我们可以打印该单元格所在的行和列,并将包含该单元格的文件复制到aa文件夹下。
以下是一个Python代码示例,实现上述操作:
``` python
import os
import shutil
import glob
import openpyxl
# 定义要查找的字符串
target_string = '\u5f20三'
# 定义要复制文件的目标文件夹
destination_folder = 'aa'
# 获取当前文件夹路径
current_directory = os.getcwd()
# 遍历所有xlsx文件
for file in glob.glob(os.path.join(current_directory, '*.xlsx')):
# 打开xlsx文件
workbook = openpyxl.load_workbook(file)
# 遍历工作表
for sheet_name in workbook.sheetnames:
worksheet = workbook[sheet_name]
# 遍历每个单元格
for row in worksheet.iter_rows():
for cell in row:
# 检查单元格是否包含目标字符串
if target_string in str(cell.value):
print(f"找到字符串'{target_string}'在 {sheet_name} 中的单元格 {cell.coordinate}")
# 复制文件到目标文件夹
source_file = os.path.join(current_directory, file)
destination_file = os.path.join(current_directory, destination_folder, os.path.basename(file))
shutil.copy(source_file, destination_file)
break
```
需要注意的是,在进行文件复制操作时,我们需要使用Python的shutil模块。其中,os.path.basename()函数可以返回文件的文件名,而os.path.join()函数可以将多个路径组合成一个路径。
### 回答2:
遍历文件夹下的所有xlsx文件,可以使用Python的os模块和glob模块来实现。首先,导入所需模块:
```python
import os
import glob
```
接下来,定义函数来遍历文件夹下的所有xlsx文件:
```python
def find_xlsx_files(folder):
xlsx_files = []
for root, dirs, files in os.walk(folder):
for file in files:
if file.endswith(".xlsx"):
xlsx_files.append(os.path.join(root, file))
return xlsx_files
```
在函数中,我们使用os.walk()方法遍历给定文件夹及其子文件夹中的所有文件,然后使用endswith(".xlsx")方法筛选出xlsx文件,并将它们的路径添加到一个列表中,最后返回该列表。
接下来,定义函数来遍历每个xlsx文件中的工作表:
```python
def find_worksheets(xlsx_file):
worksheets = pd.read_excel(xlsx_file, sheet_name=None)
return worksheets
```
在函数中,我们使用pandas库的read_excel()方法读取xlsx文件,并指定sheet_name=None参数来读取所有工作表,并将所有工作表以字典的形式返回。
然后,定义函数来遍历工作表中的每一个单元格,并查找含有"张三"的文件,并打印出名字:
```python
def find_name_in_cell(worksheets):
for name, sheet in worksheets.items():
for row in sheet.iterrows():
for value in row[1]:
if "张三" in str(value):
print(name)
```
在函数中,我们使用iterrows()方法遍历每一行,然后在每一行中遍历每一个单元格的值。如果遇到含有"张三"的值,就打印出该工作表的名字。
最后,将含有"张三"的文件复制到aa文件夹下,可以使用shutil模块的copy()方法实现:
```python
def copy_file(source, destination):
shutil.copy(source, destination)
```
在函数中,我们使用shutil.copy()方法将源文件复制到目标文件夹。
最后,可以将上述函数组合在一起,实现完整的功能:
```python
import os
import glob
import pandas as pd
import shutil
def find_xlsx_files(folder):
xlsx_files = []
for root, dirs, files in os.walk(folder):
for file in files:
if file.endswith(".xlsx"):
xlsx_files.append(os.path.join(root, file))
return xlsx_files
def find_worksheets(xlsx_file):
worksheets = pd.read_excel(xlsx_file, sheet_name=None)
return worksheets
def find_name_in_cell(worksheets):
for name, sheet in worksheets.items():
for row in sheet.iterrows():
for value in row[1]:
if "张三" in str(value):
print(name)
def copy_file(source, destination):
shutil.copy(source, destination)
folder = "文件夹路径"
destination = "aa文件夹路径"
xlsx_files = find_xlsx_files(folder)
for xlsx_file in xlsx_files:
worksheets = find_worksheets(xlsx_file)
find_name_in_cell(worksheets)
copy_file(xlsx_file, destination)
```
在代码中,需要将"文件夹路径"替换为实际的文件夹路径,将"aa文件夹路径"替换为实际的目标文件夹路径。运行代码后,会输出含有"张三"的单元格所在的工作表名字,并将含有"张三"的文件复制到目标文件夹中。
### 回答3:
通过使用Python编写脚本,可以实现遍历文件夹下的所有xlsx文件,并在每个xlsx文件中遍历工作表和每个单元格,然后查找包含"张三"的单元格并打印出姓名。最后将这些包含"张三"的文件复制到一个名为"aa"的文件夹下。
首先,需要使用`os`模块遍历文件夹下的所有文件,并筛选出xlsx文件。然后,使用`openpyxl`库打开每个xlsx文件,遍历所有工作表。接着,遍历每个工作表中的每个单元格,检查单元格值是否包含"张三",如果包含,则打印出该单元格所在的行和列,并获取对应的姓名。最后,使用`shutil`模块将这些包含"张三"的文件复制到"aa"文件夹下。
下面是一个示例的Python代码:
```python
import os
import openpyxl
import shutil
# 遍历文件夹下的所有xlsx文件
folder_path = '文件夹路径'
xlsx_files = [f for f in os.listdir(folder_path) if f.endswith('.xlsx')]
# 创建aa文件夹
aa_folder_path = 'aa文件夹路径'
if not os.path.exists(aa_folder_path):
os.makedirs(aa_folder_path)
for file in xlsx_files:
file_path = os.path.join(folder_path, file)
wb = openpyxl.load_workbook(file_path)
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
for row in sheet.iter_rows():
for cell in row:
if '张三' in str(cell.value): # 判断单元格值是否包含"张三"
print(f"找到张三!姓名:{cell.value},文件:{file},工作表:{sheet_name},单元格:{cell.coordinate}")
# 复制文件到aa文件夹下
new_file_path = os.path.join(aa_folder_path, file)
shutil.copy(file_path, new_file_path)
break
```
请替换`文件夹路径`为你要遍历的文件夹的路径,并将`aa文件夹路径`替换为你要保存包含"张三"的文件的文件夹路径。
以上代码会将包含"张三"的文件名、工作表名和单元格坐标打印输出,并将这些文件复制到aa文件夹下。
阅读全文
相关推荐
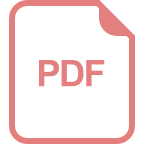
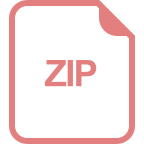
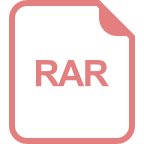















