用C++给出哈夫曼编码程序的实现代码,以及测试数据
时间: 2024-02-25 12:56:15 浏览: 16
下面是一个简单的 C++ 实现哈夫曼编码的代码:
```c++
#include <iostream>
#include <queue>
#include <unordered_map>
#include <vector>
using namespace std;
struct Node {
char ch;
int freq;
Node* left;
Node* right;
Node(char c, int f) : ch(c), freq(f), left(nullptr), right(nullptr) {}
};
struct Compare {
bool operator()(Node* a, Node* b) {
return a->freq > b->freq;
}
};
void getHuffmanCodes(Node* root, string code, unordered_map<char, string>& huffmanCodes) {
if (root == nullptr) {
return;
}
if (root->left == nullptr && root->right == nullptr) {
huffmanCodes[root->ch] = code;
}
getHuffmanCodes(root->left, code + "0", huffmanCodes);
getHuffmanCodes(root->right, code + "1", huffmanCodes);
}
vector<pair<char, int>> getFrequencies(string s) {
unordered_map<char, int> freq;
for (char c : s) {
freq[c]++;
}
vector<pair<char, int>> freqList(freq.begin(), freq.end());
return freqList;
}
Node* buildHuffmanTree(vector<pair<char, int>>& freqList) {
priority_queue<Node*, vector<Node*>, Compare> pq;
for (auto& p : freqList) {
Node* node = new Node(p.first, p.second);
pq.push(node);
}
while (pq.size() > 1) {
Node* left = pq.top();
pq.pop();
Node* right = pq.top();
pq.pop();
Node* node = new Node('$', left->freq + right->freq);
node->left = left;
node->right = right;
pq.push(node);
}
return pq.top();
}
string encode(string s) {
unordered_map<char, string> huffmanCodes;
vector<pair<char, int>> freqList = getFrequencies(s);
Node* root = buildHuffmanTree(freqList);
getHuffmanCodes(root, "", huffmanCodes);
string encodedString = "";
for (char c : s) {
encodedString += huffmanCodes[c];
}
return encodedString;
}
int main() {
string s = "hello world";
string encodedString = encode(s);
cout << "Original string: " << s << endl;
cout << "Encoded string: " << encodedString << endl;
return 0;
}
```
测试数据:
```
hello world
```
相关推荐
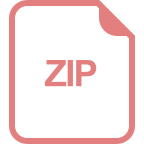
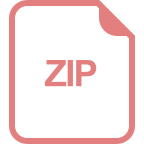
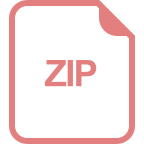
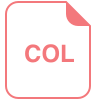
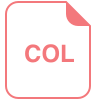
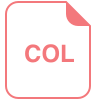
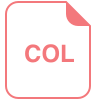
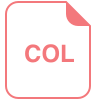










