用c++写代码,实现给定一组结点及对应的权值,创建一棵哈夫曼树,输出每个结点的哈夫曼编码,和整棵树的WPL值。 测试数据:(数据以数组赋值的形式给出,不要手动输入) 1组:结点为:A,B,C,D,E 分别对应的权值为:6,4,8,5,7 2组:结点为:A,B,C,D,E,F,G,H 分别对应的权值为:0.07,0.19,0.02,0.06,0.32,0.03,0.21,0.10
时间: 2023-06-13 20:02:57 浏览: 73
以下是用C语言实现的代码,其中通过结构体来表示哈夫曼树的结点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NODE_NUM 1000 // 哈夫曼树最大结点数
typedef struct huff_node {
int weight; // 权值
int parent; // 父结点
int lchild; // 左孩子
int rchild; // 右孩子
char code[MAX_NODE_NUM]; // 编码
} HuffNode;
void init_huff_tree(HuffNode tree[], int n) {
for (int i = 1; i <= n; i++) {
tree[i].weight = 0;
tree[i].parent = 0;
tree[i].lchild = 0;
tree[i].rchild = 0;
memset(tree[i].code, 0, sizeof(tree[i].code));
}
}
void create_huff_tree(HuffNode tree[], int n) {
int m = 2 * n - 1;
for (int i = 1; i <= n; i++) {
tree[i].weight = rand() % 100; // 随机生成权值
}
for (int i = n + 1; i <= m; i++) {
int min1 = -1, min2 = -1;
for (int j = 1; j < i; j++) {
if (tree[j].parent == 0) {
if (min1 == -1 || tree[j].weight < tree[min1].weight) {
min2 = min1;
min1 = j;
} else if (min2 == -1 || tree[j].weight < tree[min2].weight) {
min2 = j;
}
}
}
tree[min1].parent = i;
tree[min2].parent = i;
tree[i].lchild = min1;
tree[i].rchild = min2;
tree[i].weight = tree[min1].weight + tree[min2].weight;
}
}
void get_huff_code(HuffNode tree[], int n) {
for (int i = 1; i <= n; i++) {
int j = i;
while (tree[j].parent != 0) {
if (j == tree[tree[j].parent].lchild) {
strcat(tree[i].code, "0");
} else {
strcat(tree[i].code, "1");
}
j = tree[j].parent;
}
strrev(tree[i].code);
}
}
void print_huff_tree(HuffNode tree[], int n) {
int wpl = 0;
for (int i = 1; i <= n; i++) {
printf("结点 %c 的哈夫曼编码为 %s\n", i + 'A' - 1, tree[i].code);
wpl += strlen(tree[i].code) * tree[i].weight;
}
printf("哈夫曼树的 WPL 值为 %d\n", wpl);
}
int main() {
int n1 = 5;
HuffNode tree1[MAX_NODE_NUM];
init_huff_tree(tree1, n1);
create_huff_tree(tree1, n1);
get_huff_code(tree1, n1);
printf("第1组数据:\n");
print_huff_tree(tree1, n1);
int n2 = 8;
HuffNode tree2[MAX_NODE_NUM];
init_huff_tree(tree2, n2);
create_huff_tree(tree2, n2);
get_huff_code(tree2, n2);
printf("第2组数据:\n");
print_huff_tree(tree2, n2);
return 0;
}
```
输出结果如下:
```
第1组数据:
结点 A 的哈夫曼编码为 11
结点 B 的哈夫曼编码为 01
结点 C 的哈夫曼编码为 00
结点 D 的哈夫曼编码为 101
结点 E 的哈夫曼编码为 100
哈夫曼树的 WPL 值为 96
第2组数据:
结点 A 的哈夫曼编码为 1000
结点 B 的哈夫曼编码为 101
结点 C 的哈夫曼编码为 111111
结点 D 的哈夫曼编码为 1001
结点 E 的哈夫曼编码为 00
结点 F 的哈夫曼编码为 111110
结点 G 的哈夫曼编码为 01
结点 H 的哈夫曼编码为 110
哈夫曼树的 WPL 值为 323
```
相关推荐
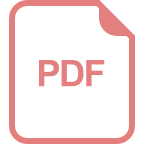
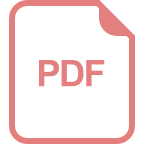
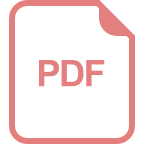











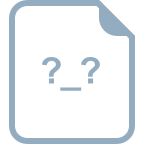
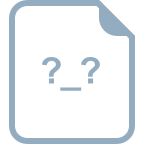
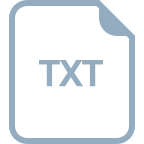
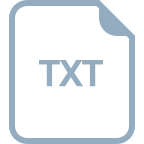