如何使用对比损失函数,请用pytorch写一下?处理文本的
时间: 2023-07-14 15:13:46 浏览: 57
对比损失函数(Contrastive Loss)是一种常见的用于计算两个样本之间距离的损失函数。在处理文本时,可以使用该损失函数来衡量两个文本之间的相似度。下面给出一个使用PyTorch实现对比损失函数的例子:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class ContrastiveLoss(nn.Module):
def __init__(self, margin=2.0):
super(ContrastiveLoss, self).__init__()
self.margin = margin
def forward(self, output1, output2, label):
euclidean_distance = F.pairwise_distance(output1, output2)
loss_contrastive = torch.mean((1-label) * torch.pow(euclidean_distance, 2) +
(label) * torch.pow(torch.clamp(self.margin - euclidean_distance, min=0.0), 2))
return loss_contrastive
# 定义模型
class TextNet(nn.Module):
def __init__(self, embedding_size, hidden_size):
super(TextNet, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_size)
self.rnn = nn.LSTM(embedding_size, hidden_size, batch_first=True)
def forward(self, x):
x = self.embedding(x)
outputs, (h_n, c_n) = self.rnn(x)
return h_n.squeeze()
# 构建数据集
train_dataset = torch.utils.data.TensorDataset(train_x1, train_x2, train_y)
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
# 初始化模型和损失函数
model = TextNet(embedding_size, hidden_size)
criterion = ContrastiveLoss()
# 定义优化器
optimizer = optim.Adam(model.parameters(), lr=lr)
# 训练模型
model.train()
for epoch in range(num_epochs):
for batch_idx, (x1, x2, y) in enumerate(train_loader):
optimizer.zero_grad()
output1 = model(x1)
output2 = model(x2)
loss = criterion(output1, output2, y)
loss.backward()
optimizer.step()
```
在这个例子中,我们首先定义了一个`ContrastiveLoss`类作为对比损失函数的实现。`forward`方法接收两个输入向量`output1`和`output2`,并计算它们之间的欧几里得距离。我们使用`torch.pow`函数来计算平方,`torch.clamp`函数来裁剪负数的值,然后取平均值作为损失函数的输出。
接下来,我们定义了一个文本分类模型`TextNet`,该模型使用`nn.Embedding`层将输入的词汇转换为嵌入向量,然后使用LSTM层进行编码。在训练过程中,我们使用`ContrastiveLoss`作为损失函数,并使用Adam优化器对模型进行优化。
最后,在每个epoch中,我们使用`train_loader`迭代训练集中的每批数据,并对模型进行训练。
相关推荐
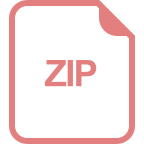
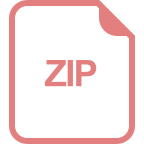
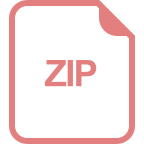
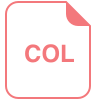
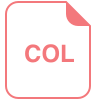
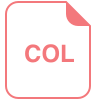
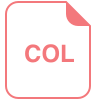










