灰度变换写一个Python代码
时间: 2023-03-26 07:01:01 浏览: 83
好的,以下是灰度变换的 Python 代码:
```python
import cv2
# 读取图片
img = cv2.imread('image.jpg')
# 灰度变换
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 显示图片
cv2.imshow('Gray Image', gray_img)
cv2.waitKey()
cv2.destroyAllWindows()
```
这段代码可以将一张彩色图片转换成灰度图片,并显示出来。
相关问题
图像增强灰度变换算法代码python
以下是一个简单的图像增强灰度变换算法的 Python 代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 灰度变换
gamma = 0.5
img_enhanced = np.power(img / float(np.max(img)), gamma)
img_enhanced = np.uint8(img_enhanced * 255)
# 显示图像
cv2.imshow('Original Image', img)
cv2.imshow('Enhanced Image', img_enhanced)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`gamma` 是灰度变换的参数,可以根据需要进行调整。
用Python写一个霍夫变换代码
以下是一个 Python 实现的霍夫变换代码示例:
```python
import numpy as np
import cv2
def hough_transform(img, theta_res=1, rho_res=1, threshold=100):
# 获取图像的高度和宽度
height, width = img.shape[:2]
# 计算极坐标中的距离
max_dist = int(np.ceil(np.sqrt(height**2 + width**2)))
rhos = np.arange(-max_dist, max_dist + 1, rho_res)
# 计算极坐标中的角度
thetas = np.deg2rad(np.arange(-90, 90, theta_res))
# 创建一个累加器数组,存储每个点的投票数
accumulator = np.zeros((len(rhos), len(thetas)), dtype=np.uint8)
# 遍历图像中的每个像素,如果像素值大于阈值,则对累加器进行投票
for y in range(height):
for x in range(width):
if img[y][x] > threshold:
for i, theta in enumerate(thetas):
rho = x*np.cos(theta) + y*np.sin(theta)
j = np.argmin(np.abs(rhos - rho))
accumulator[j][i] += 1
return accumulator, thetas, rhos
if __name__ == '__main__':
# 读取图像
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
# 进行霍夫变换
accumulator, thetas, rhos = hough_transform(img)
# 找到累加器中的最大值
max_value = np.max(accumulator)
# 设置阈值,只显示投票数大于阈值的线段
threshold = max_value * 0.5
# 在原始图像上绘制检测到的线段
for j, rho in enumerate(rhos):
for i, theta in enumerate(thetas):
if accumulator[j][i] > threshold:
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 显示结果图像
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个代码首先定义了一个 `hough_transform` 函数,该函数接受一个灰度图像作为输入,并返回一个累加器数组、角度数组和距离数组。累加器数组用于存储每个点的投票数,角度数组和距离数组用于定义霍夫空间。
然后,代码读取输入图像,并在其中运行 `hough_transform` 函数。找到累加器中的最大值后,代码使用阈值来确定哪些线段应该被绘制。最后,代码在原始图像上绘制检测到的线段并显示结果图像。
相关推荐
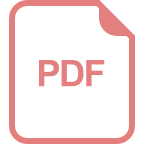
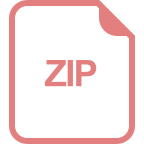












