如何用Cshape快速画三维球坐标方向图
时间: 2024-05-07 10:19:04 浏览: 145
你可以使用C#中的Unity3D引擎来快速画一个三维球坐标方向图。下面是一个简单的示例代码:
```csharp
using UnityEngine;
public class DrawSphere : MonoBehaviour
{
public GameObject directionPrefab; // 方向箭头预制体
public float radius = 1f; // 球的半径
public int subdivisions = 8; // 分段数
void Start()
{
// 绘制球面
GameObject sphere = GameObject.CreatePrimitive(PrimitiveType.Sphere);
sphere.transform.position = Vector3.zero;
sphere.transform.localScale = new Vector3(radius * 2f, radius * 2f, radius * 2f);
// 绘制坐标轴
GameObject xAxis = Instantiate(directionPrefab, Vector3.zero, Quaternion.identity);
xAxis.GetComponent<DirectionArrow>().direction = Vector3.right;
xAxis.GetComponent<DirectionArrow>().length = radius * 1.2f;
GameObject yAxis = Instantiate(directionPrefab, Vector3.zero, Quaternion.identity);
yAxis.GetComponent<DirectionArrow>().direction = Vector3.up;
yAxis.GetComponent<DirectionArrow>().length = radius * 1.2f;
GameObject zAxis = Instantiate(directionPrefab, Vector3.zero, Quaternion.identity);
zAxis.GetComponent<DirectionArrow>().direction = Vector3.forward;
zAxis.GetComponent<DirectionArrow>().length = radius * 1.2f;
// 绘制球坐标系方向箭头
for (int phi = 0; phi < subdivisions; phi++)
{
for (int theta = 0; theta < subdivisions; theta++)
{
float phif = Mathf.PI * phi / (subdivisions - 1);
float thetaf = 2f * Mathf.PI * theta / (subdivisions - 1);
Vector3 direction = new Vector3(Mathf.Sin(phif) * Mathf.Cos(thetaf), Mathf.Sin(phif) * Mathf.Sin(thetaf), Mathf.Cos(phif));
GameObject directionArrow = Instantiate(directionPrefab, Vector3.zero, Quaternion.identity);
directionArrow.GetComponent<DirectionArrow>().direction = direction;
directionArrow.GetComponent<DirectionArrow>().length = radius * 0.8f;
}
}
}
}
```
上面的代码使用了一个 `DirectionArrow` 脚本,它可以在场景中绘制一个方向箭头。下面是 `DirectionArrow` 脚本的示例实现:
```csharp
using UnityEngine;
public class DirectionArrow : MonoBehaviour
{
public Vector3 direction; // 方向
public float length = 1f; // 长度
void Start()
{
// 绘制箭头
GameObject arrow = GameObject.CreatePrimitive(PrimitiveType.Cylinder);
arrow.transform.parent = transform;
arrow.transform.localScale = new Vector3(0.05f, length, 0.05f);
arrow.transform.localPosition = new Vector3(0f, length / 2f, 0f);
arrow.GetComponent<Renderer>().material.color = Color.red;
// 绘制箭头顶点
GameObject vertex = GameObject.CreatePrimitive(PrimitiveType.Sphere);
vertex.transform.parent = transform;
vertex.transform.localScale = new Vector3(0.1f, 0.1f, 0.1f);
vertex.transform.localPosition = direction.normalized * length;
vertex.GetComponent<Renderer>().material.color = Color.red;
}
}
```
你需要将上面的代码保存为两个不同的脚本文件,并将它们附加到你的Unity场景中的一个空对象上。然后你就可以通过在 `DrawSphere` 脚本的 `directionPrefab` 字段中指定一个包含 `DirectionArrow` 组件的空对象预制体来绘制方向箭头。
阅读全文
相关推荐
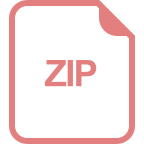
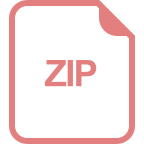


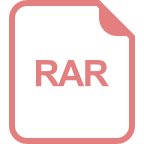
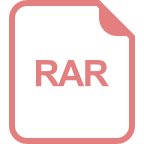
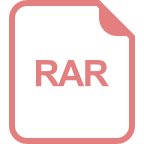
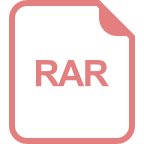
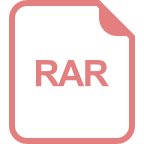
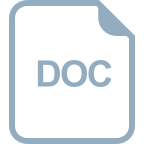






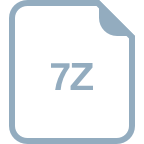
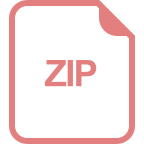