写有关stm32驱动ov5640的C语言代码
时间: 2023-08-04 11:05:23 浏览: 42
以下是基于STM32F4xx系列的驱动OV5640摄像头的示例代码,需要注意的是,这只是一个简单的示例,实际应用中需要根据具体情况进行修改和完善。
```c
#include "stm32f4xx.h"
#include "ov5640.h"
#include "delay.h"
#define OV5640_WR GPIO_Pin_15
#define OV5640_WR_H GPIO_SetBits(GPIOE, GPIO_Pin_15)
#define OV5640_WR_L GPIO_ResetBits(GPIOE, GPIO_Pin_15)
#define OV5640_RD GPIO_Pin_13
#define OV5640_RD_H GPIO_SetBits(GPIOE, GPIO_Pin_13)
#define OV5640_RD_L GPIO_ResetBits(GPIOE, GPIO_Pin_13)
#define OV5640_CS GPIO_Pin_11
#define OV5640_CS_H GPIO_SetBits(GPIOE, GPIO_Pin_11)
#define OV5640_CS_L GPIO_ResetBits(GPIOE, GPIO_Pin_11)
#define OV5640_RST GPIO_Pin_10
#define OV5640_RST_H GPIO_SetBits(GPIOE, GPIO_Pin_10)
#define OV5640_RST_L GPIO_ResetBits(GPIOE, GPIO_Pin_10)
#define OV5640_SCL GPIO_Pin_7
#define OV5640_SCL_H GPIO_SetBits(GPIOE, GPIO_Pin_7)
#define OV5640_SCL_L GPIO_ResetBits(GPIOE, GPIO_Pin_7)
#define OV5640_SDA GPIO_Pin_9
#define OV5640_SDA_H GPIO_SetBits(GPIOE, GPIO_Pin_9)
#define OV5640_SDA_L GPIO_ResetBits(GPIOE, GPIO_Pin_9)
void OV5640_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOE, ENABLE);
GPIO_InitStructure.GPIO_Pin = OV5640_WR | OV5640_RD | OV5640_CS | OV5640_RST | OV5640_SCL | OV5640_SDA;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOE, &GPIO_InitStructure);
OV5640_WR_H;
OV5640_RD_H;
OV5640_CS_H;
OV5640_RST_H;
OV5640_SCL_H;
OV5640_SDA_H;
Delay_ms(10);
OV5640_Reset();
}
void OV5640_WriteReg(uint16_t reg, uint8_t value)
{
OV5640_CS_L;
OV5640_WR_L;
OV5640_WriteByte(reg >> 8);
OV5640_WriteByte(reg & 0xFF);
OV5640_WriteByte(value);
OV5640_WR_H;
OV5640_CS_H;
}
uint8_t OV5640_ReadReg(uint16_t reg)
{
uint8_t value;
OV5640_CS_L;
OV5640_WR_L;
OV5640_WriteByte(reg >> 8);
OV5640_WriteByte(reg & 0xFF);
OV5640_RD_L;
value = OV5640_ReadByte();
OV5640_RD_H;
OV5640_CS_H;
return value;
}
void OV5640_Reset(void)
{
OV5640_RST_L;
Delay_ms(100);
OV5640_RST_H;
Delay_ms(100);
}
void OV5640_WriteByte(uint8_t data)
{
GPIO_InitTypeDef GPIO_InitStructure;
uint8_t i;
GPIO_InitStructure.GPIO_Pin = OV5640_SDA;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOE, &GPIO_InitStructure);
for (i = 0; i < 8; i++)
{
if (data & 0x80)
OV5640_SDA_H;
else
OV5640_SDA_L;
OV5640_SCL_L;
Delay_us(2);
OV5640_SCL_H;
Delay_us(2);
data <<= 1;
}
GPIO_InitStructure.GPIO_Pin = OV5640_SDA;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOE, &GPIO_InitStructure);
}
uint8_t OV5640_ReadByte(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
uint8_t i, value = 0;
GPIO_InitStructure.GPIO_Pin = OV5640_SDA;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOE, &GPIO_InitStructure);
for (i = 0; i < 8; i++)
{
OV5640_SCL_L;
Delay_us(2);
OV5640_SCL_H;
Delay_us(2);
value <<= 1;
if (GPIO_ReadInputDataBit(GPIOE, OV5640_SDA))
value |= 0x01;
}
GPIO_InitStructure.GPIO_Pin = OV5640_SDA;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOE, &GPIO_InitStructure);
return value;
}
```
以上代码中,需要注意的是:
1. OV5640_Init()函数用于初始化OV5640摄像头,其中包括GPIO初始化和OV5640_Reset()函数调用。
2. OV5640_WriteReg()函数用于向OV5640写寄存器,其中包括寄存器地址和数据。
3. OV5640_ReadReg()函数用于从OV5640读取寄存器的值,其中包括寄存器地址。
4. OV5640_Reset()函数用于复位OV5640摄像头。
5. OV5640_WriteByte()函数用于向OV5640写一个字节,其中包括数据。
6. OV5640_ReadByte()函数用于从OV5640读一个字节的数据。
需要注意的是,以上代码只是一个简单的示例,实际应用中需要根据具体情况进行修改和完善。另外,还需要根据OV5640摄像头的寄存器手册进行编程,以实现各种功能。
相关推荐
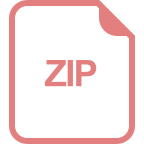














