. 输出若干个学生成绩中的最高分.要求用指针函数实现c语言
时间: 2023-12-25 21:29:26 浏览: 167
好的,以下是用指针函数实现输出若干个学生成绩中的最高分的C语言代码:
```
#include <stdio.h>
// 定义函数,返回类型为float指针类型,参数为float类型的数组和数组长度
float* max(float arr[], int len) {
// 定义指针变量,初始化为数组第一个元素的地址
float* p = &arr[0];
// 遍历数组,找到最大值的地址
for (int i = 1; i < len; i++) {
if (arr[i] > *p) {
p = &arr[i];
}
}
// 返回最大值的地址
return p;
}
int main() {
float scores[] = {89.5, 76.3, 92.0, 85.6, 78.2}; // 定义学生成绩数组
int len = sizeof(scores) / sizeof(scores[0]); // 计算数组长度
float* p = max(scores, len); // 调用函数,得到最大值的地址
printf("最高分为:%.1f", *p); // 输出最高分
return 0;
}
```
注意,这里返回的是最高分的地址,需要用 `*p` 取出最高分的值进行输出。
相关问题
c语言.输入4个学生的成绩,计算并输出其中的最高分。
在C语言中,你可以通过以下步骤来实现这个功能:
1. 首先,你需要定义一个变量来存储最高分数,通常初始化为数组中的第一个元素(假设学生们的初始成绩都是0)。
```c
float maxScore = students[0];
```
2. 然后,遍历给定的四个学生成绩,如果发现有比当前`maxScore`更高的分数,就更新`maxScore`。
```c
for (int i = 1; i < 4; i++) {
if (students[i] > maxScore) {
maxScore = students[i];
}
}
```
3. 最后,使用`printf`函数将最高分输出到屏幕上。
```c
printf("最高分为: %.2f\n", maxScore);
```
完整的代码示例:
```c
#include <stdio.h>
int main() {
float students[4]; // 假设输入的学生分数
float maxScore = students[0];
printf("请输入第1个学生的成绩: ");
scanf("%f", &students[0]);
printf("请输入第2个学生的成绩: ");
scanf("%f", &students[1]);
printf("请输入第3个学生的成绩: ");
scanf("%f", &students[2]);
printf("请输入第4个学生的成绩: ");
scanf("%f", &students[3]);
for (int i = 1; i < 4; i++) {
if (students[i] > maxScore) {
maxScore = students[i];
}
}
printf("最高分为: %.2f\n", maxScore);
return 0;
}
```
C语言用函数实现统计出若干个学生的平均成绩,最高分以及得最高分的人数
好的,以下是C语言函数实现统计出学生的平均成绩、最高分和得最高分的人数的代码:
```c
#include <stdio.h>
void score_analysis(float scores[], int n, float* ave_score, float* max_score, int* max_count) {
float sum = 0, max = scores[0];
int count = 1;
for(int i = 0; i < n; i++) {
sum += scores[i];
if(scores[i] > max) {
max = scores[i];
count = 1;
} else if(scores[i] == max) {
count++;
}
}
*ave_score = sum / n;
*max_score = max;
*max_count = count;
}
int main() {
float scores[] = { 85, 76, 92, 88, 90 };
int n = sizeof(scores) / sizeof(scores[0]);
float ave_score, max_score;
int max_count;
score_analysis(scores, n, &ave_score, &max_score, &max_count);
printf("平均成绩:%.2f\n", ave_score);
printf("最高分:%.2f\n", max_score);
printf("得最高分的人数:%d\n", max_count);
return 0;
}
```
在这个例子中,我们定义了一个名为score_analysis的函数,该函数接收一个包含学生成绩的数组,数组大小和三个整数指针的参数。然后,函数计算平均分、最高分和得最高分的人数,并将结果存储在提供的指针变量中。
在main函数中,我们创建了一个包含5个学生成绩的数组,并将其作为score_analysis函数的输入。接下来,我们打印出结果。
希望这个例子能够回答你的问题。如果你有任何其他问题,都可以向我提出。
阅读全文
相关推荐
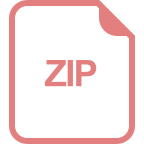
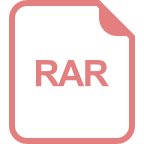













