编写一个程序来实现二叉搜索算法。如果找不到,则返回“无”
时间: 2023-06-02 16:08:00 浏览: 65
好的,我可以帮你编写二叉搜索算法。以下是 Python 代码示例:
```python
class Node:
def __init__(self, val=None):
self.val = val
self.left = None
self.right = None
def binary_search(root, target):
if root is None:
return None
if root.val == target:
return root
elif root.val > target:
return binary_search(root.left, target)
else:
return binary_search(root.right, target)
```
例如,要在以下二叉树中查找值为 4 的节点:
```
5
/ \
2 7
/ \
1 3
/
4
```
可以这样调用函数:
```python
root = Node(5)
root.left = Node(2)
root.right = Node(7)
root.left.left = Node(1)
root.left.right = Node(3)
root.left.left.left = Node(4)
result = binary_search(root, 4)
if result:
print("找到了:", result.val)
else:
print("无")
```
输出:
```
找到了: 4
```
阅读全文
相关推荐
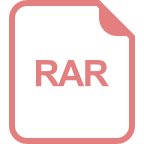
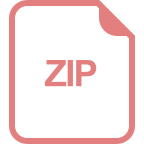
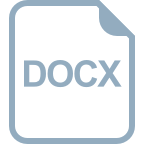
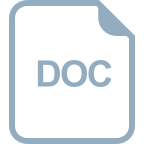
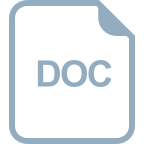
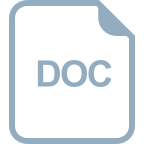
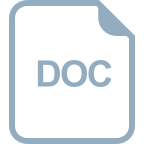
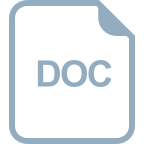
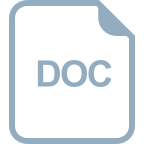
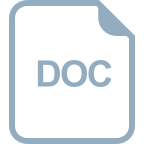


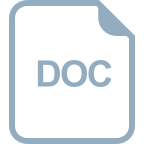
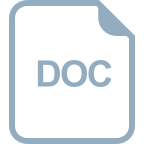
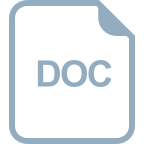
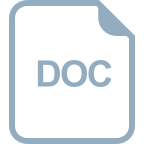
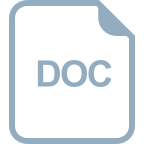
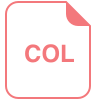