如何改使得以下程序一直运行include<unistd.h> #include<stdio.h> int main() { int pid; pid=fork(); pid=fork(); if(pid>0)fork(); printf("hello!\n"); sleep(30); return 0; }
时间: 2024-01-12 09:05:08 浏览: 72
可以使用一个死循环来让程序一直运行,例如:
```c
#include <unistd.h>
#include <stdio.h>
int main() {
int pid;
pid = fork();
pid = fork();
if (pid > 0) {
fork();
}
while (1) {
printf("hello!\n");
sleep(30);
}
return 0;
}
```
这样程序会一直输出"hello!",并且每次输出之后都会暂停30秒钟。需要注意的是,由于程序没有任何终止条件,因此需要手动中断程序来停止它的执行。
相关问题
#include <unistd.h> #include <string.h> #include <stdio.h> #include <sys/stat.h> #include <fcntl.h>
`#include <unistd.h>`是一个C语言的头文件,它包含了一些与系统调用相关的函数和常量。其中一些常用的函数包括`fork()`、`exec()`和`pipe()`等。`<string.h>`是另一个C语言的头文件,它包含了一些与字符串操作相关的函数,例如`strcpy()`和`strlen()`等。`<stdio.h>`是标准输入输出的头文件,它包含了一些与输入输出相关的函数,例如`printf()`和`scanf()`等。`<sys/stat.h>`是一个系统状态的头文件,它包含了一些与文件状态相关的函数和常量,例如`stat()`和`chmod()`等。`<fcntl.h>`是文件控制的头文件,它包含了一些与文件操作相关的函数和常量,例如`open()`和`close()`等。
以下是一个简单的示例代码,演示了如何使用这些头文件中的函数:
```c
#include <unistd.h>
#include <string.h>
#include <stdio.h>
#include <sys/stat.h>
#include <fcntl.h>
int main() {
// 使用unistd.h中的函数
int pid = fork();
if (pid == 0) {
// 子进程
printf("This is child process.\n");
} else if (pid > 0) {
// 父进程
printf("This is parent process.\n");
} else {
// 出错
perror("fork");
return 1;
}
// 使用string.h中的函数
char str1[10] = "Hello";
char str2[10];
strcpy(str2, str1);
printf("Copied string: %s\n", str2);
// 使用stdio.h中的函数
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
// 使用sys/stat.h和fcntl.h中的函数
int fd = open("file.txt", O_RDONLY);
struct stat fileStat;
fstat(fd, &fileStat);
printf("File size: %ld bytes\n", fileStat.st_size);
close(fd);
return 0;
}
```
这段代码演示了如何使用`fork()`函数创建子进程,使用`strcpy()`函数复制字符串,使用`scanf()`函数读取用户输入的数字,以及使用`open()`函数打开文件并使用`fstat()`函数获取文件大小。
例1. 编写如下程序,保存为forkt1.c。 #include <sys/types.h> #include <unistd.h> #include <stdio.h> void main() {
这是一个使用fork函数创建子进程的C程序。程序的主要功能是创建一个子进程,并在子进程中输出一条信息。
代码如下:
```c
#include <sys/types.h>
#include <unistd.h>
#include <stdio.h>
int main()
{
pid_t pid;
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork failed\n");
return 1;
} else if (pid == 0) {
printf("This is the child process\n");
} else {
printf("This is the parent process\n");
}
return 0;
}
```
这个程序首先包含了一些头文件,然后定义了一个主函数`main()`。在`main()`函数中,首先声明了一个`pid_t`类型的变量`pid`,用于保存fork函数的返回值。
接下来,调用了fork函数,将返回值赋给`pid`变量。fork函数会创建一个新的进程,如果创建成功,则在父进程中返回子进程的PID,在子进程中返回0。如果创建失败,则返回-1。
然后,通过判断pid的值,可以确定当前是在父进程还是子进程中。如果pid小于0,则说明fork失败,输出错误信息。如果pid等于0,则说明当前是在子进程中,输出一条信息"This is the child process"。如果pid大于0,则说明当前是在父进程中,输出一条信息"This is the parent process"。
最后,主函数返回0,表示程序正常结束。
阅读全文
相关推荐
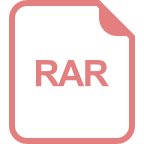
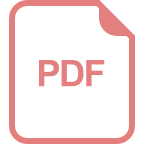
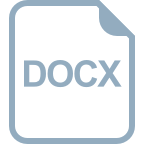













