#include <sys/types.h> #include <unistd.h> #include <stdio.h> #include <stdlib.h> int main(){ pid_t pid; char *message; int n; pid=fork(); if (pid<0){ perror("fork failed"); exit(1); } if(pid==0){ message="This is the children\n"; n=3; } else { message="This is the parent\n"; n=6; } for(;n>0;n--){ printf(message); // sleep(1); } return 0; } 修改程序,在子进程中给变量n赋值6,父进程中给变量n赋值3,请分析程序运行的结果。
时间: 2024-03-25 08:36:37 浏览: 29
修改后的程序如下:
```
#include <sys/types.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
pid_t pid;
char *message;
int n;
pid = fork();
if (pid < 0) {
perror("fork failed");
exit(1);
}
if (pid == 0) {
message = "This is the child\n";
n = 6;
}
else {
message = "This is the parent\n";
n = 3;
}
for (; n > 0; n--) {
printf(message);
}
return 0;
}
```
在子进程中,将 `n` 的值赋为6,在父进程中,将 `n` 的值赋为3。
运行程序,输出的结果如下:
```
This is the parent
This is the parent
This is the parent
This is the child
This is the child
This is the child
This is the child
This is the child
This is the child
```
可以看到,现在输出的顺序是先输出父进程的信息,再输出子进程的信息。这是因为在父进程中,`n` 的值为3,所以在 `for` 循环中只会执行三次,而在子进程中,`n` 的值为6,所以在 `for` 循环中会执行六次。因此,子进程的输出会比父进程的输出多。
相关推荐
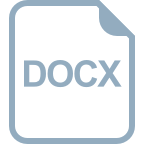
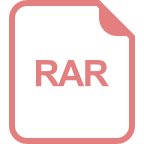
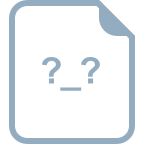














