#include <sys/types.h> #include <unistd.h> #include <stdio.h> #include <stdlib.h> int main(void){ pid_t pid; char *message; int x; pid = fork(); if (pid < 0) { perror("fork failed"); exit(1); } if (pid == 0) { message = "This is the child\n"; x = 0; } else { message = "This is the parent\n"; x = 10; //sleep(2); } printf("%s I'm %d, x=%d,my father is:%d\n",message,x,getpid(),getppid()); return 0; }//main修改以上代码
时间: 2024-03-23 14:40:16 浏览: 90
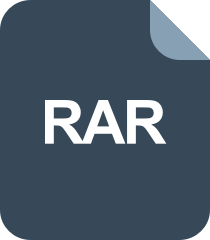
用c语言实现的逆序文本程序
这段代码是一个简单的进程创建程序,它通过调用fork()函数创建了一个子进程,然后在父进程和子进程中分别输出不同的消息和变量值。
如果我们想要让父进程等待子进程执行完毕后再继续执行,我们可以使用wait()函数或者waitpid()函数来实现。
以下是修改后的代码,它在父进程中等待子进程执行完毕后再输出消息:
```c
#include <sys/types.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/wait.h> // 添加头文件
int main(void){
pid_t pid;
char *message;
int x;
int status; // 添加变量
pid = fork();
if (pid < 0) {
perror("fork failed");
exit(1);
}
if (pid == 0) {
message = "This is the child\n";
x = 0;
} else {
message = "This is the parent\n";
x = 10;
wait(&status); // 父进程等待子进程执行完毕
}
printf("%s I'm %d, x=%d,my father is:%d\n", message, x, getpid(), getppid());
return 0;
}
```
在父进程中,我们添加了一个wait()函数,它会等待子进程执行完毕并返回子进程的状态信息。由于我们只需要等待子进程执行完毕,所以我们可以将status变量定义为一个简单的整型变量。
阅读全文
相关推荐
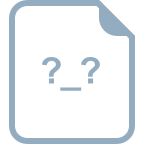
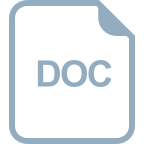














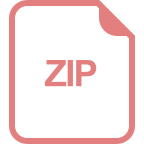