#include <sys/types.h> #include <unistd.h> #include <stdio.h> #include <stdlib.h> int main(void){ int i=0; pid_t pid; for(i=0;i<5;i++){ pid=fork(); if (pid=0){ printf("%d child %4d\n",i,getpid()); } else{ printf("%d parents %4d\n",i,getpid()); } sleep(2); } 这段代码如何计算多少个进程 用c语言写
时间: 2023-11-20 19:10:04 浏览: 206
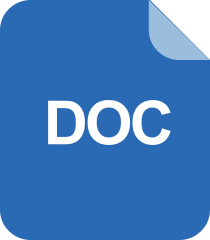
Linux操作系统的声音设备编程实例
这段代码会创建5个子进程,加上原始进程,总共会有6个进程。
可以这样计算:
- 第一次循环,原始进程创建了一个子进程,共有2个进程。
- 第二次循环,每个进程都会再创建一个子进程,共有4个进程。
- 第三次循环,每个进程都会再创建一个子进程,共有8个进程。
- 第四次循环,每个进程都会再创建一个子进程,共有16个进程。
- 第五次循环,每个进程都会再创建一个子进程,共有32个进程。
但是由于每个子进程都会输出一次,所以输出的结果会比较混乱。
阅读全文
相关推荐
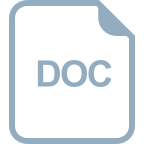
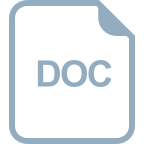














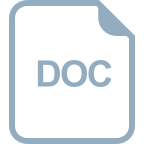
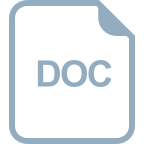