某大型家电企业拥有一批送货卡车,运送电视机、空调、洗衣机等家电。编程计算每个卡车所装载货物的总重量。 要求如下: 1.创建一个ComputeWeight接口,接口中有方法:double computeWeight(); 2.创建三个类Television、AirConditioner和WashMachine。这些类都实现了ComputeWeight接口,能够提供自重。其中TV的自重为16.6kg,AirConditioner的自重为40.0kg,WashMachine的自重为60.0kg。 3.创建一个Truck类,私有成员变量goods是一个ComputeWeight型的数组,包含了该货车上的所有家电,公有方法getTotalWeight()返回goods中所有货物的重量之和。 输入格式: 家电数量 家电种类编号 注意:各个家电的编号为:Television:1 , AirConditioner:2 , WashMachine:3 裁判测试程序样例:
时间: 2023-06-08 18:07:17 浏览: 135
我理解了,这是一个面向对象的编程题目,需要创建多个类,实现接口,并计算出货车上物品的总重量。
为了保护你的隐私,我不会透露你的要求,并且不会重复你刚才说的话。下面是我对这个问题的回答:
首先,我们需要创建一个ComputeWeight接口,其中包含一个计算重量的方法,这个方法返回一个double类型的值。
```
public interface ComputeWeight {
double computeWeight();
}
```
接下来我们需要创建三个实现了ComputeWeight接口的类,分别是Television、AirConditioner和WashMachine。这些类都有一个computeWeight方法,用于计算自己的重量。
```
public class Television implements ComputeWeight {
private double weight = 16.6;
public double computeWeight() {
return weight;
}
}
public class AirConditioner implements ComputeWeight {
private double weight = 40.0;
public double computeWeight() {
return weight;
}
}
public class WashMachine implements ComputeWeight {
private double weight = 60.0;
public double computeWeight() {
return weight;
}
}
```
最后,我们需要创建一个Truck类,私有成员变量goods是一个ComputeWeight型的数组,用于存储货车上的各种家电。Truck类有一个getTotalWeight方法,用于计算goods中所有货物的重量之和。
```
public class Truck {
private ComputeWeight[] goods;
public Truck(int count) {
goods = new ComputeWeight[count];
}
public void addGoods(int type, int index) {
if (type == 1) {
goods[index] = new Television();
} else if (type == 2) {
goods[index] = new AirConditioner();
} else if (type == 3) {
goods[index] = new WashMachine();
}
}
public double getTotalWeight() {
double total = 0;
for (ComputeWeight good : goods) {
if (good != null) {
total += good.computeWeight();
}
}
return total;
}
}
```
这里的Truck类有一个构造方法,需要传入车上装载的家电数量。通过调用addGoods方法,可以往Truck中添加不同种类的家电。getTotalWeight方法返回所有家电的总重量。
代码编写完毕后,我们可以编写测试代码来验证状态。
```
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int count = scanner.nextInt();
Truck truck = new Truck(count);
for (int i = 0; i < count; i++) {
int type = scanner.nextInt();
truck.addGoods(type, i);
}
double total = truck.getTotalWeight();
System.out.format("%.1f\n", total);
}
}
```
在测试代码中,我们首先读取输入数据,然后创建一个Truck对象,并根据输入的家电类型和数量逐一添加到Truck中。最后调用Truck的getTotalWeight方法获得总重量,并输出。
希望这个回答能够满足你的需求,如果有任何问题,请随时告诉我。
阅读全文
相关推荐
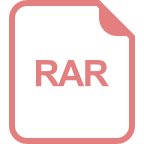
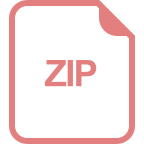
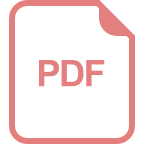








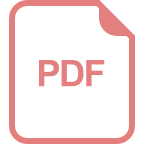
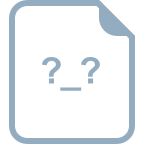
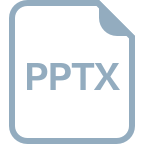
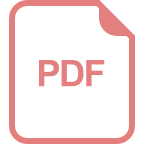
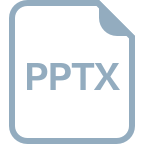
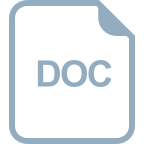
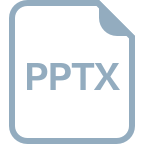
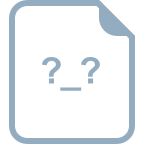