用Java实现一个超级玛丽小游戏,实现基本的游戏操作
时间: 2023-06-10 17:09:08 浏览: 124
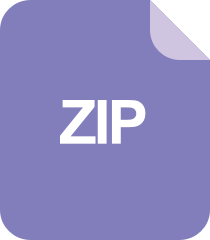
超级玛丽游戏GUI(源码+论文+视频齐全)
好的,我可以为您提供一个简单的Java代码示例,实现基本的超级玛丽小游戏操作。
在这个小游戏中,玩家需要控制超级玛丽来躲避障碍物并收集金币。以下是代码示例:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class SuperMario extends JPanel implements KeyListener, Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int GROUND = 500;
private static final int SPEED = 10;
private boolean isRunning = true;
private boolean isJumping = false;
private int x = 0;
private int y = GROUND;
private int jumpHeight = 100;
private int jumpCount = 0;
private Image mario = new ImageIcon("mario.png").getImage();
private Image background = new ImageIcon("background.png").getImage();
private Image coin = new ImageIcon("coin.png").getImage();
private int coinX = 700;
private int coinY = GROUND - 50;
public static void main(String[] args) {
JFrame frame = new JFrame("Super Mario");
frame.setSize(WIDTH, HEIGHT);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.setResizable(false);
SuperMario game = new SuperMario();
frame.add(game);
frame.setVisible(true);
new Thread(game).start();
}
public void paint(Graphics g) {
g.drawImage(background, 0, 0, null);
g.drawImage(mario, x, y, null);
g.drawImage(coin, coinX, coinY, null);
g.setColor(Color.WHITE);
g.drawString("Press SPACE to jump", 50, 50);
}
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_SPACE && !isJumping) {
isJumping = true;
}
}
public void keyReleased(KeyEvent e) {}
public void keyTyped(KeyEvent e) {}
public void run() {
while (isRunning) {
if (isJumping) {
y -= SPEED;
jumpCount++;
if (jumpCount == jumpHeight) {
isJumping = false;
jumpCount = 0;
}
} else if (y < GROUND) {
y += SPEED;
}
if (coinX < 0) {
coinX = 800;
}
coinX -= SPEED;
if (x + mario.getWidth(null) > coinX
&& x < coinX + coin.getWidth(null)
&& y + mario.getHeight(null) > coinY
&& y < coinY + coin.getHeight(null)) {
coinX = 800;
}
repaint();
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
这个示例代码实现了以下功能:
- 通过键盘控制超级玛丽跳跃
- 超级玛丽会在跳跃时向上移动,并在达到一定高度后自动落下
- 超级玛丽会在落地时停止移动
- 超级玛丽会在接触到金币时收集金币
您可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
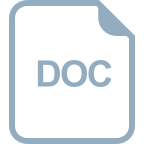
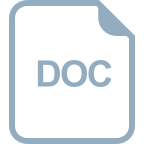




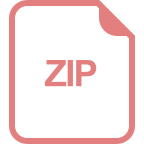
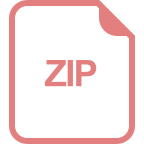
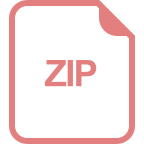
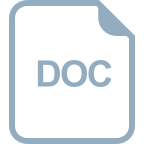
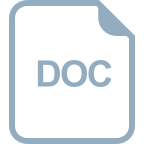
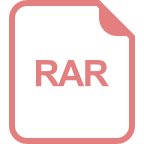
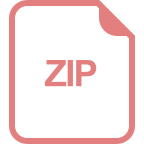
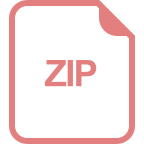
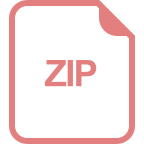
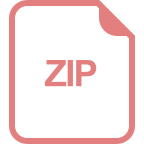