c++利用分支界限法实现旅行商问题
时间: 2023-08-02 14:06:53 浏览: 75
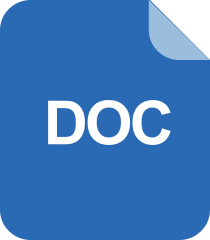
用分支限界法解决旅行商问题

旅行商问题是一个经典的组合优化问题,它的目标是在访问所有城市并返回起点的情况下,寻找最短的旅行路线。分支界限法是一种广泛应用于求解组合优化问题的方法,下面是使用C++实现旅行商问题的分支界限算法:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 城市数量
const int MAXN = 10;
// 旅行商问题类
class TSP {
public:
TSP() {}
~TSP() {}
void init(); // 初始化城市距离矩阵
void solve(); // 求解旅行商问题
private:
int n; // 城市数量
int d[MAXN][MAXN]; // 城市距离矩阵
int best_cost; // 最优解的花费
vector<int> best_path; // 最优解的路径
// 状态类
class State {
public:
State(int _cost, vector<int> _path) {
cost = _cost;
path = _path;
}
~State() {}
bool operator<(const State& s) const {
return cost > s.cost;
}
int cost; // 花费
vector<int> path; // 路径
};
// 分支界限法求解
void branch_and_bound();
};
// 初始化城市距离矩阵
void TSP::init() {
n = 5;
int dis[MAXN][MAXN] = {
{ 0, 2, 3, 4, 5 },
{ 2, 0, 3, 4, 5 },
{ 3, 3, 0, 4, 5 },
{ 4, 4, 4, 0, 5 },
{ 5, 5, 5, 5, 0 }
};
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
d[i][j] = dis[i][j];
}
}
}
// 求解旅行商问题
void TSP::solve() {
best_cost = 1e9;
best_path.clear();
branch_and_bound();
cout << "最优解的花费为:" << best_cost << endl;
cout << "最优解的路径为:";
for (int i = 0; i < n; i++) {
cout << best_path[i] << " ";
}
cout << endl;
}
// 分支界限法求解
void TSP::branch_and_bound() {
priority_queue<State> q; // 优先队列
vector<int> init_path;
init_path.push_back(0);
State init_state(0, init_path);
q.push(init_state);
while (!q.empty()) {
State s = q.top();
q.pop();
if (s.cost >= best_cost) continue; // 剪枝
if (s.path.size() == n) { // 更新最优解
s.cost += d[s.path[n - 1]][0];
if (s.cost < best_cost) {
best_cost = s.cost;
best_path = s.path;
}
continue;
}
for (int i = 0; i < n; i++) {
bool flag = true;
for (int j = 0; j < s.path.size(); j++) {
if (i == s.path[j]) {
flag = false;
break;
}
}
if (flag) {
vector<int> new_path = s.path;
new_path.push_back(i);
State new_state = State(s.cost + d[s.path.back()][i], new_path);
q.push(new_state);
}
}
}
}
int main() {
TSP tsp;
tsp.init();
tsp.solve();
return 0;
}
```
上述代码中,`TSP` 类封装了旅行商问题的求解过程,其中 `init()` 函数用于初始化城市距离矩阵,`solve()` 函数用于求解旅行商问题,`branch_and_bound()` 函数实现了分支界限法求解过程。
在 `branch_and_bound()` 函数中,使用了优先队列来维护候选状态,每次取出花费最小的状态进行扩展。在扩展状态时,如果当前状态的花费已经超过了当前最优解的花费,则进行剪枝,不再继续扩展。如果当前状态已经访问了所有城市,则将当前状态作为最优解进行更新。否则,将当前状态的每个未访问城市作为下一步的选择,生成新的状态,并加入优先队列中。
阅读全文
相关推荐
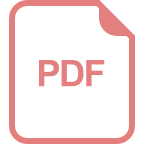



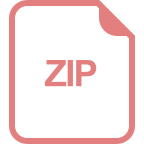
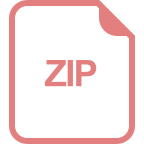
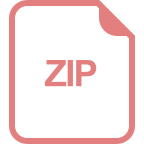