pandas .loc[row_indexer,col_indexer]
时间: 2023-02-07 20:03:50 浏览: 193
pandas 的 .loc 属性用于选择 DataFrame 中的行和列。row_indexer 参数指定要选择的行的索引,col_indexer 参数指定要选择的列的索引。例如:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# 选择第 1 行,第 'A' 列和第 'C' 列
df.loc[1, ['A', 'C']]
```
输出:
```
A 2
C 8
Name: 1, dtype: int64
```
相关问题
pandas 出现此错误A value is trying to be set on a copy of a slice from a DataFrame. Try using .loc[row_indexer,col_indexer] = value instead
这个错误通常出现在使用 Pandas 进行切片操作后进行赋值时。为了避免这个错误,建议使用 `.loc` 或 `.iloc` 来进行赋值操作。
这是因为对 DataFrame 进行切片操作会返回一个视图(view)而不是副本(copy),因此对视图进行赋值可能会影响到原始 DataFrame。为了明确指定要修改的数据位置,可以使用 `.loc` 或 `.iloc` 方法。
以下是一个示例代码:
```python
import pandas as pd
# 创建示例数据
data = {'col1': ['80123', '80456', '70123', '80234', '90567']}
df = pd.DataFrame(data)
# 使用 .loc 进行赋值
df.loc[df['col1'].str.startswith('80'), 'col1'] = '90' + df['col1'].str[2:]
print(df)
```
输出结果如下:
```
col1
0 90123
1 90456
2 70123
3 90234
4 90567
```
在这个示例中,我们使用了 `.loc` 来定位以 '80' 开头的行,并对该位置进行赋值操作。我们通过 `'90' + df['col1'].str[2:]` 来获取替换后的值。这样可以避免出现上述错误。
SettingWithCopyWarning: A value is trying to be set on a copy of a slice from a DataFrame. Try using .loc[row_indexer,col_indexer] = value instead See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy tnl_data['Gender_Label'] = gender_label_enc.fit_transform(tnl_data['Gender']) D:\学习\商务智能\大作业\参考模型\预测代码.py:217: SettingWithCopyWarning: A value is trying to be set on a copy of a slice from a DataFrame. Try using .loc[row_indexer,col_indexer] = value instead See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy tnl_data['Marital_Label'] = marital_label_enc.fit_transform(tnl_data['MaritalStatus'])
这个警告是由 Pandas 提供的,它通常是在使用链式索引时出现的。在上述代码中,可能是 `tnl_data` 是一个 DataFrame 的切片,而不是对原始 DataFrame 的引用,所以在对该切片进行修改时,会出现警告。
为了避免这个警告,可以使用 `.loc` 或 `.iloc` 来对 DataFrame 进行索引和修改,而不是使用链式索引。例如,可以将代码修改为:
```python
tnl_data.loc[:, 'Gender_Label'] = gender_label_enc.fit_transform(tnl_data['Gender'])
tnl_data.loc[:, 'Marital_Label'] = marital_label_enc.fit_transform(tnl_data['MaritalStatus'])
```
这样就可以避免警告了。另外,你也可以通过设置 Pandas 的选项来禁用这个警告,但不建议这样做。
阅读全文
相关推荐
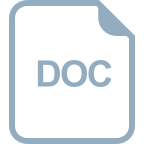
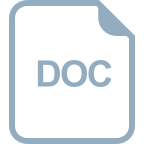
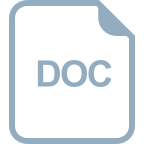




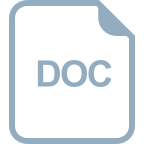
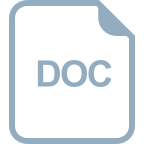
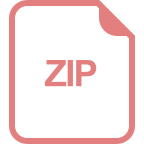
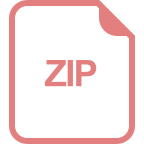
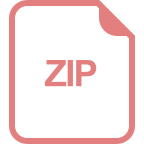
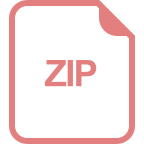
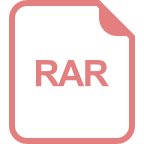
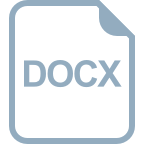
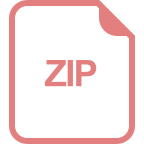