c++ 给出一棵二叉树的前序和中序遍历,求它的后序遍历
时间: 2023-07-22 07:05:07 浏览: 108
假设二叉树的前序遍历为preorder,中序遍历为inorder,那么可以使用递归的方式求解后序遍历。具体来说,找到前序遍历中的第一个节点,即为根节点,然后在中序遍历中找到根节点所在的位置,将中序遍历分成左子树和右子树两部分,递归求解左子树和右子树的后序遍历,最后将根节点加入到后序遍历的末尾即可。
以下是 C++ 代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
vector<int> postorder(vector<int>& preorder, vector<int>& inorder) {
vector<int> res;
if (preorder.empty() || inorder.empty()) {
return res;
}
int rootVal = preorder[0]; // 前序遍历的第一个节点为根节点
int rootIndex = 0;
while (inorder[rootIndex] != rootVal) {
rootIndex++;
}
vector<int> leftInorder(inorder.begin(), inorder.begin() + rootIndex); // 左子树的中序遍历
vector<int> rightInorder(inorder.begin() + rootIndex + 1, inorder.end()); // 右子树的中序遍历
preorder.erase(preorder.begin()); // 删除已经使用过的根节点
vector<int> leftPostorder = postorder(preorder, leftInorder); // 左子树的后序遍历
vector<int> rightPostorder = postorder(preorder, rightInorder); // 右子树的后序遍历
res.insert(res.end(), leftPostorder.begin(), leftPostorder.end()); // 将左子树的后序遍历添加到结果中
res.insert(res.end(), rightPostorder.begin(), rightPostorder.end()); // 将右子树的后序遍历添加到结果中
res.push_back(rootVal); // 最后将根节点加入到结果中
return res;
}
int main() {
vector<int> preorder = {1, 2, 4, 5, 3, 6, 7};
vector<int> inorder = {4, 2, 5, 1, 6, 3, 7};
vector<int> postorder = postorder(preorder, inorder);
for (int i = 0; i < postorder.size(); i++) {
cout << postorder[i] << " ";
}
return 0;
}
```
输出结果为:4 5 2 6 7 3 1
阅读全文
相关推荐
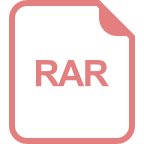
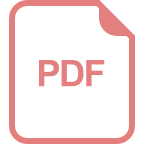
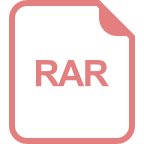












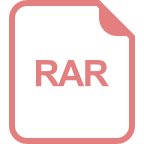
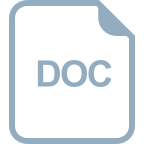