python A 星算法 如何实现
时间: 2024-01-01 21:04:40 浏览: 122
在Python中实现A*算法,可以按照以下步骤进行:
1. 定义节点类Node,包含节点坐标、FCost值、GCost值、HCost值和父节点信息等属性,以及计算FCost值和更新父节点等方法。
```python
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.f_cost = 0
self.g_cost = 0
self.h_cost = 0
self.parent = None
def calculate_f_cost(self):
self.f_cost = self.g_cost + self.h_cost
def update_parent(self, parent_node, cost):
self.parent = parent_node
self.g_cost = parent_node.g_cost + cost
self.calculate_f_cost()
```
2. 定义A*算法函数,接收起点和终点坐标作为参数,返回最短路径。
```python
def a_star(start, end):
open_list = [Node(start[0], start[1])]
closed_list = []
while open_list:
# 选取FCost值最小的节点
current_node = min(open_list, key=lambda node: node.f_cost)
# 将该节点从开启列表中移除
open_list.remove(current_node)
# 将该节点加入关闭列表中
closed_list.append(current_node)
# 如果当前节点是终点,则返回最短路径
if current_node.x == end[0] and current_node.y == end[1]:
path = []
while current_node:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
# 遍历当前节点的相邻节点
for neighbor_node in get_neighbors(current_node):
# 如果该节点已经在关闭列表中,则跳过
if neighbor_node in closed_list:
continue
# 计算从起点到该节点的GCost值
cost = get_move_cost(current_node, neighbor_node)
# 如果该节点不在开启列表中,则加入开启列表
if neighbor_node not in open_list:
neighbor_node.update_parent(current_node, cost)
open_list.append(neighbor_node)
# 如果该节点已经在开启列表中,则更新其父节点和GCost值
else:
if current_node.g_cost + cost < neighbor_node.g_cost:
neighbor_node.update_parent(current_node, cost)
# 如果开启列表为空,则无法到达终点,返回None
return None
```
3. 定义获取相邻节点和移动代价的函数,根据实际情况进行实现。
```python
def get_neighbors(node):
neighbors = []
# 获取左侧节点
if node.x > 0:
neighbors.append(Node(node.x - 1, node.y))
# 获取右侧节点
if node.x < max_x:
neighbors.append(Node(node.x + 1, node.y))
# 获取上方节点
if node.y > 0:
neighbors.append(Node(node.x, node.y - 1))
# 获取下方节点
if node.y < max_y:
neighbors.append(Node(node.x, node.y + 1))
return neighbors
def get_move_cost(current_node, neighbor_node):
# 计算从当前节点到相邻节点的移动代价
# 可以根据实际情况进行修改
if current_node.x == neighbor_node.x or current_node.y == neighbor_node.y:
return 1
else:
return 1.414
```
需要注意的是,以上代码中的max_x和max_y都是地图的宽度和高度,需要根据实际情况进行修改。此外,还需要预先将起点和终点坐标转换为Node对象,并设置起点的GCost值和HCost值。
阅读全文
相关推荐
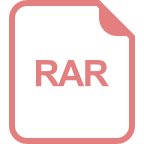
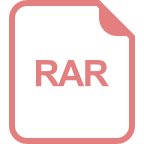
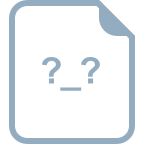
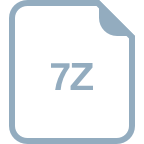
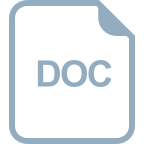
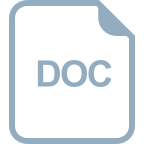
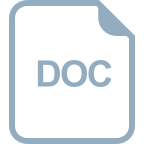







