定义一个图书类(Book),该类的数据成员有:书名(bookname)、作者(authors)、页数(pages)、出版社(publishing_house)、价格(price),要求如下 : (1)图书的所有属性都是私有的,数据成员的类型根据实际情况来确定; (2)给该类添加相应的getXxx、setXxx函数(例如:char * getBookname()、void setBookname(char * bk))来获取、设置该类的私有成员; (3)给该类添加适当的构造函数、析构函数来初始化、清理对象; (4)在main()函数定义两本图书: 《C++程序设计》,谭浩强编著,485页,清华大学出版社,36.00元 《吹牛大王历险记》,拉斯伯等编著,149页,天津人民出版社,12.80元 然后将这两个对象的成员信息输出到屏幕上。
时间: 2023-06-25 14:07:28 浏览: 234
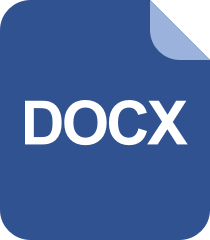
WCF开发实战教程

下面是一个实现上述要求的示例代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class Book {
private:
string bookname;
string authors;
int pages;
string publishing_house;
double price;
public:
Book() {
bookname = "";
authors = "";
pages = 0;
publishing_house = "";
price = 0;
}
Book(string bkname, string auth, int pg, string pubhouse, double prc) {
bookname = bkname;
authors = auth;
pages = pg;
publishing_house = pubhouse;
price = prc;
}
~Book() {}
string getBookname() const {
return bookname;
}
void setBookname(string bkname) {
bookname = bkname;
}
string getAuthors() const {
return authors;
}
void setAuthors(string auth) {
authors = auth;
}
int getPages() const {
return pages;
}
void setPages(int pg) {
pages = pg;
}
string getPublishingHouse() const {
return publishing_house;
}
void setPublishingHouse(string pubhouse) {
publishing_house = pubhouse;
}
double getPrice() const {
return price;
}
void setPrice(double prc) {
price = prc;
}
};
int main() {
Book book1("C++程序设计", "谭浩强编著", 485, "清华大学出版社", 36.00);
Book book2("吹牛大王历险记", "拉斯伯等编著", 149, "天津人民出版社", 12.80);
cout << "书名:" << book1.getBookname() << endl;
cout << "作者:" << book1.getAuthors() << endl;
cout << "页数:" << book1.getPages() << endl;
cout << "出版社:" << book1.getPublishingHouse() << endl;
cout << "价格:" << book1.getPrice() << "元" << endl;
cout << endl;
cout << "书名:" << book2.getBookname() << endl;
cout << "作者:" << book2.getAuthors() << endl;
cout << "页数:" << book2.getPages() << endl;
cout << "出版社:" << book2.getPublishingHouse() << endl;
cout << "价格:" << book2.getPrice() << "元" << endl;
return 0;
}
```
输出结果如下:
```
书名:C++程序设计
作者:谭浩强编著
页数:485
出版社:清华大学出版社
价格:36元
书名:吹牛大王历险记
作者:拉斯伯等编著
页数:149
出版社:天津人民出版社
价格:12.8元
```
阅读全文
相关推荐
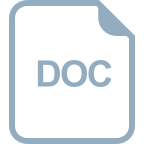
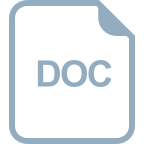















