解释一下compareSheetDetailList.stream().map(CompareSheetDetail::getPriceSheetDetail).map(PriceSheetDetail::getId).collect(Collectors.toSet())
时间: 2023-06-05 13:07:19 浏览: 97
这是一段Java 8中使用StreamAPI对比较表的详细列表进行操作的代码。具体操作是先通过map方法将CompareSheetDetailList中的每个CompareSheetDetail对象的PriceSheetDetail转换出来,再通过map方法将得到的PriceSheetDetail对象中的id属性转换出来,最后通过collect(Collectors.toSet())将所有id去重并存储到Set集合中。简单来说,这段代码的作用是获取CompareSheetDetailList中所有PriceSheetDetail的id并去重。
相关问题
list.stream().map(LocationEntity::getId).collect(Collectors.toSet())解释一下作用
这段代码的作用是将一个 List 集合中的 LocationEntity 对象的 id 属性提取出来,并将提取的 id 放入一个 Set 集合中。具体解释如下:
- `list.stream()`:将 List 集合转换为 Stream 流,以便进行后续的操作。
- `map(LocationEntity::getId)`:通过 `map` 操作,将流中的每个 LocationEntity 对象映射为其对应的 id 属性值。`LocationEntity::getId` 表示获取 LocationEntity 对象的 id 属性值。
- `collect(Collectors.toSet())`:将流中的元素收集到一个 Set 集合中。`Collectors.toSet()` 表示将流中的元素收集到一个新的 HashSet 实例中,保证元素的唯一性。
综上所述,这段代码的作用是将 List 集合中每个 LocationEntity 对象的 id 属性值提取出来,并将提取的 id 放入一个 Set 集合中,最终得到一个不重复的 id 集合。
我需要通过hutool工具批量更新数据库中的字段,根据id来更新,id是这样获取的final List<Integer> notPerformed = entityList.stream().map(VppResponseEventEntity::getId).collect(Collectors.toList());
可以使用Hutool的Db类来操作数据库,具体步骤如下:
1. 引入Hutool的Db依赖,Maven坐标如下:
```xml
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-db</artifactId>
<version>${hutool.version}</version>
</dependency>
```
注意替换`${hutool.version}`为具体的版本号。
2. 获取数据库连接:
```java
// 假设使用MySQL数据库,连接信息如下
String url = "jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8";
String username = "root";
String password = "123456";
// 获取数据库连接
DbUtil db = DbUtil.use();
db.setDriver("com.mysql.cj.jdbc.Driver");
db.setUrl(url);
db.setUser(username);
db.setPassword(password);
```
3. 构造SQL语句和参数:
```java
// 构造SQL语句
String sql = "UPDATE vpp_response_event SET field1=?, field2=? WHERE id=?";
// 构造参数
List<Object[]> params = new ArrayList<>();
for (VppResponseEventEntity entity : entityList) {
Object[] param = new Object[3];
param[0] = entity.getField1();
param[1] = entity.getField2();
param[2] = entity.getId();
params.add(param);
}
```
这里假设需要更新表`vpp_response_event`中的`field1`和`field2`字段,根据`id`来更新,其中`entityList`是一个包含实体对象的列表,需要根据其中的属性值构造SQL语句和参数。
4. 执行更新操作:
```java
// 批量执行更新操作
int[] results = db.batch(sql, params.toArray(new Object[0][]));
```
这里使用了DbUtil的`batch`方法来批量执行更新操作,返回值是一个整数数组,表示每条SQL语句更新的记录数。
完整代码如下:
```java
// 假设使用MySQL数据库,连接信息如下
String url = "jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8";
String username = "root";
String password = "123456";
// 获取数据库连接
DbUtil db = DbUtil.use();
db.setDriver("com.mysql.cj.jdbc.Driver");
db.setUrl(url);
db.setUser(username);
db.setPassword(password);
// 构造SQL语句
String sql = "UPDATE vpp_response_event SET field1=?, field2=? WHERE id=?";
// 构造参数
List<Object[]> params = new ArrayList<>();
for (VppResponseEventEntity entity : entityList) {
Object[] param = new Object[3];
param[0] = entity.getField1();
param[1] = entity.getField2();
param[2] = entity.getId();
params.add(param);
}
// 批量执行更新操作
int[] results = db.batch(sql, params.toArray(new Object[0][]));
```
阅读全文
相关推荐
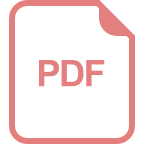
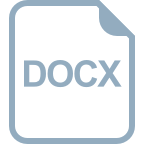
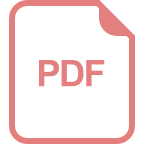













