char数组和char* 类型如何去除前后空格
时间: 2023-11-30 12:02:40 浏览: 549
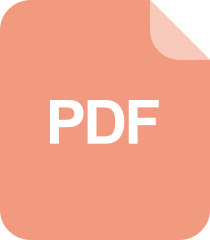
Shell中去除字符串前后空格的方法
可以使用以下方法去除char数组和char*类型的前后空格:
1. 对于char数组,可以使用标准库函数`strncpy`将其复制到一个新的char数组中,然后使用`isspace`函数判断是否为空格,从而去除前面和后面的空格。
示例代码:
```c++
#include <cstring>
#include <cctype>
void trim(char* str) {
char* end = str + strlen(str) - 1;
// 去除末尾空格
while (end >= str && isspace(*end))
end--;
*(end + 1) = '\0';
// 去除开头空格
while (*str && isspace(*str))
str++;
strcpy(str, end + 2 - strlen(str));
}
```
2. 对于char*类型,可以使用相同的方法,但需要先将其复制到一个新的char数组中。
示例代码:
```c++
#include <cstring>
#include <cctype>
char* trim(const char* str) {
char* newStr = strdup(str);
char* end = newStr + strlen(newStr) - 1;
// 去除末尾空格
while (end >= newStr && isspace(*end))
end--;
*(end + 1) = '\0';
// 去除开头空格
while (*newStr && isspace(*newStr))
newStr++;
strcpy(newStr, end + 2 - strlen(newStr));
return newStr;
}
```
注意,这里使用了`strdup`函数来复制char*类型的字符串,因此需要在使用完后手动释放内存。
阅读全文
相关推荐














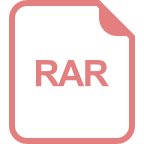
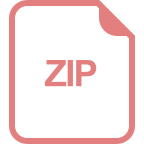