react 18.2.0 refs 代码实列
时间: 2024-05-14 22:16:04 浏览: 96
在 React 18.2.0 中,Refs 可以使用 `createRef()` 方法创建,然后通过 `ref` 属性将其附加到组件上,以便在组件中访问 DOM 元素或组件实例。以下是一个示例:
```jsx
import React, { createRef } from 'react';
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = createRef(); // 创建一个 Ref
}
componentDidMount() {
console.log(this.myRef.current); // 访问 Ref 中的 DOM 元素
}
render() {
return <div ref={this.myRef}>Hello World</div>; // 将 Ref 附加到组件上
}
}
```
在上面的示例中,我们首先使用 `createRef()` 方法创建了一个 Ref,然后在组件的 `render()` 方法中使用 `ref` 属性将其附加到一个 `div` 元素上。在组件的 `componentDidMount()` 生命周期钩子中,我们可以通过 `this.myRef.current` 访问该 `div` 元素。这是一个简单的示例,你也可以将 Ref 附加到其他组件上。
相关问题
react 18.2.0 class函數 refs 代码实列
以下是一个使用 React 18.2.0 和 class 函数组件的代码示例,其中包含使用 refs 的实例:
```
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.textInput = React.createRef();
}
componentDidMount() {
this.textInput.current.focus();
}
render() {
return (
<div>
<input type="text" ref={this.textInput} />
</div>
);
}
}
export default MyComponent;
```
在上面的示例中,我们创建了一个名为 `MyComponent` 的 class 函数组件,并在 constructor 中使用 `React.createRef()` 方法来创建一个名为 `textInput` 的 ref 对象。在 `componentDidMount()` 生命周期方法中,我们使用 `this.textInput.current.focus()` 方法来将焦点设置在输入框上。最后,我们在 render 方法中将 ref 对象传递给输入框元素,以便我们可以在其他地方使用它。
当组件挂载时,`componentDidMount()` 方法将被调用,并且我们可以使用 `this.textInput.current` 引用到输入框元素。在这个示例中,我们使用 `focus()` 方法将焦点设置为输入框。
这只是一个简单的示例,但它演示了如何使用 React 18.2.0 中的 class 函数组件和 refs。
react 18.2.0 class函數 refs 代码实列 最优
以下是一个使用React 18.2.0及以上版本的class函数和refs的最优示例代码:
```
import React from 'react';
class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
componentDidMount() {
this.myRef.current.focus();
}
render() {
return (
<div>
<input type="text" ref={this.myRef} />
</div>
);
}
}
export default ExampleComponent;
```
在此示例中,我们使用`React.createRef()`创建一个`myRef`引用,然后在`componentDidMount()`生命周期方法中,我们将焦点设置在该输入框上。在渲染函数中,我们将`myRef`引用传递给输入框元素的`ref`属性。
这种方法是React 18及以上版本中使用refs的最优方法,因为它使用最新的`React.createRef()`API,并且将`ref`保存在实例变量中而不是字符串中。
阅读全文
相关推荐
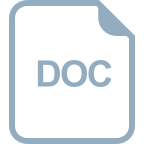
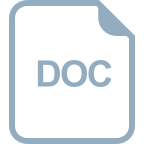
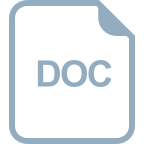
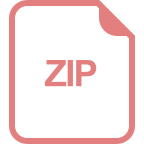

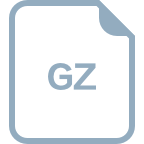
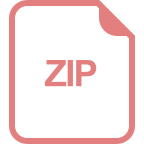
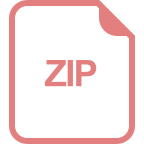
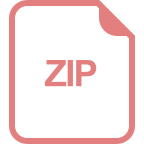
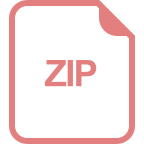
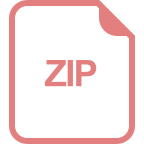
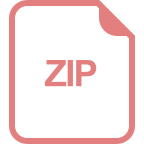
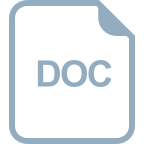



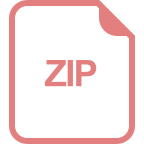