设计一个父类Person类,包含数据成员:身份证号、姓名、性别、出生日 期,要求可以完成以下操作: 1)可以从键盘输入基本信息; 2)可以输出基本信息到屏幕; 3)可以修改基本信息; 4)可以比较两个人的年纪大小; 5)可以计算出两个人出生日期相差的天数。 再设计一个子类Student类,在父类的基础上新增数据成员:学号、语文、 数学、英语成绩 ,要求可以完成以下操作: 1)可以从键盘输入学生信息; 2)可以输出学生信息到屏幕; 3)可以修改学生基本信息; 4)可以计算每位学生的语数英平均分; 5)可以按多个学生的平均分从高到低排序,并输出到屏幕; 6)可以比较两个学生年纪大小; 7)可以计算出两个学生出生日期相差的天数。
时间: 2023-05-23 08:02:20 浏览: 137
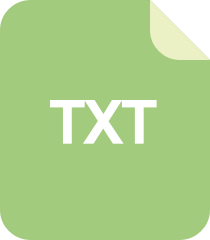
设计一个人类person,包括人员编号和姓名等数据成员以及相关的成员函数
好的,下面是我的回答:
实现父类Person类:
```python
class Person:
def __init__(self, id, name, gender, birthdate):
self.id = id
self.name = name
self.gender = gender
self.birthdate = birthdate
def input_info(self):
self.id = input("请输入身份证号:")
self.name = input("请输入姓名:")
self.gender = input("请输入性别:")
self.birthdate = input("请输入出生日期(格式:yyyy-mm-dd):")
def output_info(self):
print("身份证号:", self.id)
print("姓名:", self.name)
print("性别:", self.gender)
print("出生日期:", self.birthdate)
def modify_info(self):
self.id = input("请输入身份证号:")
self.name = input("请输入姓名:")
self.gender = input("请输入性别:")
self.birthdate = input("请输入出生日期(格式:yyyy-mm-dd):")
def compare_age(self, other):
this_year = int(self.birthdate.split("-")[0])
other_year = int(other.birthdate.split("-")[0])
if this_year < other_year:
return -1
elif this_year > other_year:
return 1
else:
return 0
def days_diff(self, other):
this_date = datetime.datetime.strptime(self.birthdate, "%Y-%m-%d")
other_date = datetime.datetime.strptime(other.birthdate, "%Y-%m-%d")
return abs((this_date - other_date).days)
```
实现子类Student类:
```python
class Student(Person):
def __init__(self, id, name, gender, birthdate, student_id, chinese_score, math_score, english_score):
super().__init__(id, name, gender, birthdate)
self.student_id = student_id
self.chinese_score = chinese_score
self.math_score = math_score
self.english_score = english_score
def input_info(self):
super().input_info()
self.student_id = input("请输入学号:")
self.chinese_score = float(input("请输入语文成绩:"))
self.math_score = float(input("请输入数学成绩:"))
self.english_score = float(input("请输入英语成绩:"))
def output_info(self):
super().output_info()
print("学号:", self.student_id)
print("语文成绩:", self.chinese_score)
print("数学成绩:", self.math_score)
print("英语成绩:", self.english_score)
def modify_info(self):
super().modify_info()
self.student_id = input("请输入学号:")
self.chinese_score = float(input("请输入语文成绩:"))
self.math_score = float(input("请输入数学成绩:"))
self.english_score = float(input("请输入英语成绩:"))
def average_score(self):
return (self.chinese_score + self.math_score + self.english_score) / 3
def compare_age(self, other):
if isinstance(other, Student):
return super().compare_age(other)
else:
return "无法比较"
def days_diff(self, other):
if isinstance(other, Student):
return super().days_diff(other)
else:
return "无法计算"
```
lua closure factory 完整代码:
```lua
function newCounter()
local count = 0
return function()
count = count + 1
return count
end
end
c1 = newCounter()
print(c1()) -- 1
print(c1()) -- 2
print(c1()) -- 3
c2 = newCounter()
print(c2()) -- 1
print(c1()) -- 4
```
中文加密的问题应该是指对中文进行加密和解密的算法,比如像凯撒密码一样对中文字符进行移位操作。实现起来比较麻烦,这里我就不提供代码了,可以自行搜索相关算法。
阅读全文
相关推荐
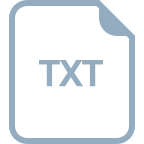
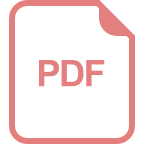















