在window系统下利用vscode开发平台实现驱动通讯协议为Canopen应用层DS -301V4.02的伺服驱动电机,请用C++语言编写,要求写一个机器人底盘运动控制代码。
时间: 2024-05-03 22:18:26 浏览: 196
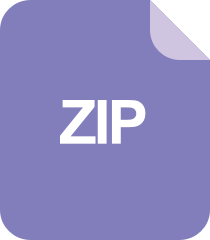
CanOpen 通讯 DS301-V4.02英文版
这是一个比较复杂的项目,需要涉及到很多知识点。以下是一些可能有用的参考资料和思路:
1. 了解Canopen协议和DS-301V4.02标准,掌握Canopen通讯的基本流程和数据格式。
2. 确定所使用的Canopen接口和控制器,例如使用Peak-System的PCAN-USB接口和Beckhoff的EtherCAT控制器。
3. 在vscode中创建一个C++项目,添加Canopen通讯库和驱动电机控制库。
4. 实现Canopen通讯的初始化、数据发送和接收功能,包括PDO和SDO等数据类型的处理。
5. 根据驱动电机的规格和控制方式,编写相应的控制代码,实现机器人底盘的运动控制,例如前进、后退、转弯等功能。
6. 测试和调试代码,确保机器人能够按照预期的方式运动。
以下是一些示例代码,供参考:
```cpp
#include <iostream>
#include <pcan.h> // Canopen通讯库
#include "servo_driver.h" // 驱动电机控制库
using namespace std;
// 定义CAN通讯参数
TPCANHandle h = PCAN_USBBUS1;
TPCANMsg msg;
TPCANTimestamp timestamp;
// 定义驱动电机对象和控制参数
ServoDriver driver(h, 1);
double velocity = 0; // 当前速度
double acceleration = 1; // 加速度
double deceleration = 1; // 减速度
// 初始化Canopen通讯
void initCanopen() {
TPCANStatus status;
status = CAN_Initialize(h, PCAN_BAUD_1M, 0, 0, 0);
if (status != PCAN_ERROR_OK) {
cout << "CAN initialization failed: " << status << endl;
exit(1);
}
}
// 发送Canopen数据
void sendCanopenData(int node_id, int index, int subindex, unsigned char* data, int len) {
TPCANStatus status;
msg.ID = 0x600 + node_id;
msg.LEN = len + 2;
msg.DATA[0] = 0x40 + ((index & 0xFF) >> 8);
msg.DATA[1] = index & 0xFF;
msg.DATA[2] = subindex;
for (int i = 0; i < len; i++) {
msg.DATA[3 + i] = data[i];
}
status = CAN_Write(h, &msg);
if (status != PCAN_ERROR_OK) {
cout << "CAN write failed: " << status << endl;
exit(1);
}
}
// 接收Canopen数据
bool receiveCanopenData(int node_id, int index, int subindex, unsigned char* data, int len) {
TPCANStatus status;
int count = 0;
while (count < 10) { // 最多等待1秒钟
status = CAN_Read(h, &msg, ×tamp);
if (status == PCAN_ERROR_OK && msg.ID == 0x580 + node_id && msg.LEN == len + 4 &&
msg.DATA[0] == ((index & 0xFF) >> 8) && msg.DATA[1] == (index & 0xFF) &&
msg.DATA[2] == subindex) {
for (int i = 0; i < len; i++) {
data[i] = msg.DATA[3 + i];
}
return true;
}
count++;
}
return false;
}
// 启动驱动电机
void startMotor() {
driver.setControlword(0x06); // 完全使能
}
// 停止驱动电机
void stopMotor() {
driver.setControlword(0x00); // 完全失能
velocity = 0;
}
// 控制机器人前进
void moveForward() {
velocity += acceleration;
if (velocity > MAX_VELOCITY) {
velocity = MAX_VELOCITY;
}
driver.setTargetVelocity(velocity);
}
// 控制机器人后退
void moveBackward() {
velocity -= acceleration;
if (velocity < -MAX_VELOCITY) {
velocity = -MAX_VELOCITY;
}
driver.setTargetVelocity(velocity);
}
// 控制机器人转弯
void turn(double angle) {
double radius = WHEELBASE / tan(angle);
double vl = velocity * (radius - WHEELBASE / 2) / radius;
double vr = velocity * (radius + WHEELBASE / 2) / radius;
driver.setTargetVelocity(vl, vr);
}
// 控制机器人停止
void stop() {
double sign = velocity > 0 ? 1 : -1;
while (abs(velocity) > 0.1) {
velocity -= sign * deceleration;
driver.setTargetVelocity(velocity);
}
stopMotor();
}
int main() {
initCanopen();
startMotor();
moveForward();
sleep(2);
turn(PI / 4);
sleep(1);
moveBackward();
sleep(2);
turn(-PI / 4);
sleep(1);
stop();
return 0;
}
```
需要注意的是,以上代码仅供参考,实际的实现可能会因为硬件平台和驱动电机规格的不同而有所变化。
阅读全文
相关推荐
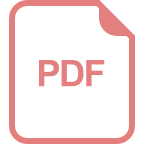
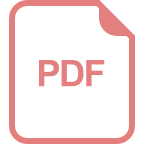














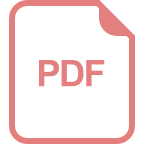