设计class:student,根据你的需求分析,定义数据成员和成员函数,类型、数量和功能无限制,要求: class中需要至少分别定义1个static和const成员; 至少3种构造函数:不带参数的构造函数,带参数有默认值的构造函数,拷贝构造函数以及你需要的其他构造函数; 析构函数:自定义析构函数; 使用友元函数,实现合适的功能; 编写测试程序main(),测试你定义的class:student中的所有功能
时间: 2023-10-23 20:14:13 浏览: 41
以下是一个参考的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
// 定义静态变量和常量
static int num_students;
const string school_name;
// 构造函数
Student() : school_name("ABC School") {
num_students++;
cout << "调用无参构造函数" << endl;
}
Student(string name, int age = 18, float score = 0.0) : name(name), age(age), score(score), school_name("ABC School"){
num_students++;
cout << "调用带参数的构造函数" << endl;
}
Student(const Student &other) : name(other.name), age(other.age), score(other.score), school_name("ABC School") {
num_students++;
cout << "调用拷贝构造函数" << endl;
}
// 析构函数
~Student() {
num_students--;
cout << "调用析构函数" << endl;
}
// 成员函数
void set_name(string name) {
this->name = name;
}
void set_age(int age) {
this->age = age;
}
void set_score(float score) {
this->score = score;
}
void study() {
cout << name << "正在学习" << endl;
}
// 友元函数
friend void print_student_info(const Student &stu);
private:
string name;
int age;
float score;
};
// 定义静态变量
int Student::num_students = 0;
// 友元函数
void print_student_info(const Student &stu) {
cout << "姓名:" << stu.name << endl;
cout << "年龄:" << stu.age << endl;
cout << "成绩:" << stu.score << endl;
}
int main() {
Student s1; // 调用无参构造函数
s1.set_name("张三");
s1.set_age(20);
s1.set_score(90.5);
Student s2("李四"); // 调用带参数的构造函数
s2.set_age(21);
s2.set_score(88.5);
Student s3(s1); // 调用拷贝构造函数
print_student_info(s1);
print_student_info(s2);
print_student_info(s3);
cout << "学生人数:" << Student::num_students << endl;
return 0;
}
```
程序输出:
```
调用无参构造函数
调用带参数的构造函数
调用拷贝构造函数
姓名:张三
年龄:20
成绩:90.5
姓名:李四
年龄:21
成绩:88.5
姓名:张三
年龄:20
成绩:90.5
学生人数:3
调用析构函数
调用析构函数
调用析构函数
```
相关推荐
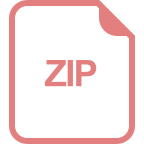
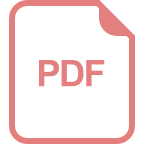














