C语言求二叉树从根节点到给定节点路径
时间: 2024-05-01 08:20:56 浏览: 109
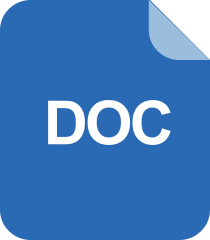
求二叉树根到给定节点的路径设计报告.doc
假设二叉树的每个节点定义如下:
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
```
其中 `val` 表示节点的值,`left` 和 `right` 分别表示左子树和右子树。
以下是求从根节点到给定节点的路径的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
int getPath(struct TreeNode* root, struct TreeNode* target, int* path, int index) {
if (root == NULL) {
return 0;
}
path[index] = root->val;
if (root == target) {
return 1;
}
if (getPath(root->left, target, path, index + 1) ||
getPath(root->right, target, path, index + 1)) {
return 1;
}
return 0;
}
int main() {
// 创建二叉树
struct TreeNode* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
// 求从根节点到给定节点的路径
int targetVal = 5;
struct TreeNode* target = root->left->right;
int path[100];
int found = getPath(root, target, path, 0);
if (found) {
printf("Path from root to target:\n");
for (int i = 0; i < found; ++i) {
printf("%d ", path[i]);
}
printf("\n");
} else {
printf("Target node not found in the tree.\n");
}
// 释放二叉树的内存
free(root->left->right);
free(root->left->left);
free(root->right->right);
free(root->right->left);
free(root->left);
free(root->right);
free(root);
return 0;
}
```
上面的 `getPath` 函数实现了求从根节点到给定节点的路径,它的参数依次为:
- `root`:当前子树的根节点;
- `target`:要查找的目标节点;
- `path`:保存路径的数组;
- `index`:当前节点在路径中的下标。
当找到目标节点时,返回 1,否则返回 0。在递归调用左右子树之前,将当前节点的值保存到路径数组中,并且将下标加 1。如果左右子树中有一颗包含目标节点,就返回 1,表示找到了目标节点。如果左右子树都不包含目标节点,就返回 0,表示没有找到目标节点。
在主函数中,我们创建了一个二叉树,并且指定要查找值为 5 的节点。然后调用 `getPath` 函数求解路径,最后打印出路径数组。注意,在程序结束前,需要释放二叉树的内存,避免内存泄漏。
阅读全文
相关推荐

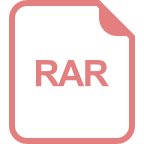
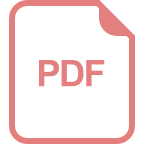












