用Java写代码:输入一串英文句子,统计句子里面出现的相同单词个数并对这些单词相应出现的次数进行排序
时间: 2024-02-17 10:01:09 浏览: 171
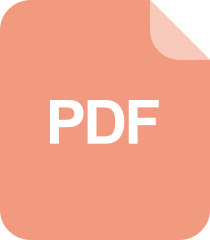
Java编程实现统计一个字符串中各个字符出现次数的方法
可以使用Java中的HashMap来实现单词计数和排序。
以下是示例代码:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
// 输入一串英文句子
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一串英文句子:");
String sentence = scanner.nextLine();
// 将句子按照空格分割成单词
String[] words = sentence.split("\\s+");
// 使用HashMap来保存单词计数
Map<String, Integer> wordCountMap = new HashMap<>();
for (String word : words) {
// 统计单词出现的次数
Integer count = wordCountMap.get(word);
if (count == null) {
count = 0;
}
wordCountMap.put(word, count + 1);
}
// 对单词计数进行排序
List<Map.Entry<String, Integer>> wordCountList = new ArrayList<>(wordCountMap.entrySet());
Collections.sort(wordCountList, new Comparator<Map.Entry<String, Integer>>() {
@Override
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o2.getValue().compareTo(o1.getValue());
}
});
// 输出结果
for (Map.Entry<String, Integer> entry : wordCountList) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
```
输入示例:
```
请输入一串英文句子:the quick brown fox jumps over the lazy dog
```
输出示例:
```
the:2
over:1
quick:1
brown:1
fox:1
jumps:1
lazy:1
dog:1
```
阅读全文
相关推荐
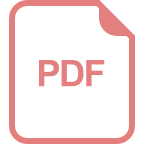















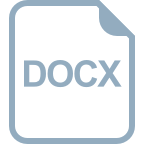