编辑一个函数,求字符 ch 在字符串 s 中出现的所有位置,并将位置保存在一个新数组中。设 s 长度不大于 50,并写出 main 函数来调用,打印 ch 的出现次数和所有位置信息
时间: 2024-03-26 15:38:50 浏览: 37
好的,下面是一个实现此功能的 C 函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void find_char_positions(char *s, char ch, int *positions, int *count) {
*count = 0;
int len = strlen(s);
for (int i = 0; i < len; i++) {
if (s[i] == ch) {
positions[*count] = i;
(*count)++;
}
}
}
int main() {
char s[51];
char ch;
int positions[50];
int count = 0;
printf("请输入一个字符串:");
fgets(s, 51, stdin);
s[strcspn(s, "\n")] = '\0'; // 去掉最后的换行符
printf("请输入要查找的字符:");
scanf("%c", &ch);
find_char_positions(s, ch, positions, &count);
printf("%c出现的次数为%d,位置为:", ch, count);
for (int i = 0; i < count; i++) {
printf("%d ", positions[i]);
}
printf("\n");
return 0;
}
```
这个函数使用了指针参数来返回字符出现的位置和出现次数。在 main 函数中,先读入字符串和要查找的字符,然后调用 `find_char_positions` 函数来查找字符出现的位置。最后打印出出现次数和所有位置信息。
注意,这里假设输入的字符串不超过 50 个字符,所以数组的大小设置为 50。如果实际情况可能超过 50,需要对代码进行修改。
阅读全文
相关推荐
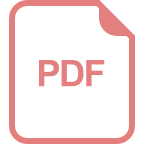

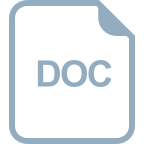













