二叉树深度代码c语言
时间: 2023-08-02 18:07:21 浏览: 51
以下是二叉树深度的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 计算二叉树深度的函数
int maxDepth(struct TreeNode* root) {
if (root == NULL) {
return 0;
} else {
int left_depth = maxDepth(root->left);
int right_depth = maxDepth(root->right);
return left_depth > right_depth ? left_depth + 1 : right_depth + 1;
}
}
// 测试
int main() {
// 构造一棵二叉树
struct TreeNode *root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = NULL;
root->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->val = 3;
root->right->left = NULL;
root->right->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->right->val = 4;
root->right->right->left = NULL;
root->right->right->right = NULL;
// 计算二叉树深度
int depth = maxDepth(root);
printf("The depth of the binary tree is: %d\n", depth);
return 0;
}
```
这个程序中,我们定义了一个 `TreeNode` 结构体,用于表示二叉树的节点。`maxDepth` 函数是递归计算二叉树深度的函数,它的返回值是左子树和右子树深度中的较大值加 1。在 `main` 函数中,我们构造了一棵二叉树并计算了它的深度。运行程序,输出结果为:
```
The depth of the binary tree is: 3
```
相关推荐
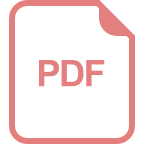
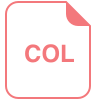
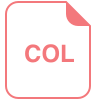
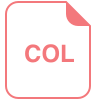











