unet pytorch
时间: 2023-09-21 11:05:52 浏览: 213
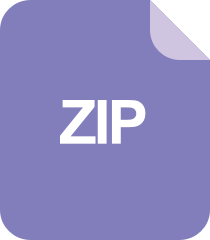
unet-pytorch
Sure, I can answer your question about UNet PyTorch. UNet is a popular convolutional neural network architecture commonly used in biomedical image segmentation tasks. PyTorch is an open-source machine learning framework that provides tools for building and training neural networks, including UNet. Is there anything specific you would like to know about UNet PyTorch?
阅读全文
相关推荐
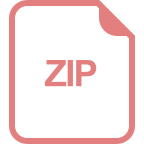
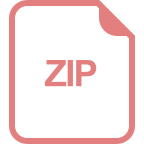
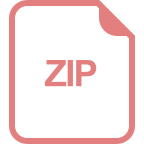



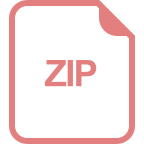
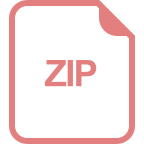
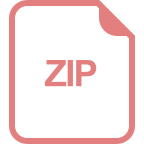
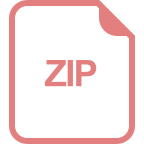




