以顺序表方式存储,编写程序实现: (1)对图书表的创建(初始创建5本书); (2)分别在5和11的位置上插入两本书:(011,python程序设计,23)和(012,数据结构,55); (3)删除第2本书; (3)获取第3本书的信息并打印; (4)打印出最贵的书和最便宜的书; (5)打印经过上述操作后,所剩的图书信息。 long id; char name[20]; float price; 例如:测试输入: 001 book1 78 002 book2 54 003 book3 45 004 book4 98 005 book5 76 006 book6 12 007 book7 14 008 book8 17 009 book9 58 010 book10 10 测试输出: 第3本书是:4 book4 98 最大值:book4 最小值:book10 1 book1 78 3 book3 45 4 book4 98 11 python程序设计 23 5 book5 76 6 book6 12 7 book7 14 8 book8 17 9 book9 58 12 数据结构 55 10 book10 10
时间: 2024-03-21 15:44:18 浏览: 16
好的,以下是根据您提供的输入数据编写的程序代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 20 // 最大书名长度
// 定义图书结构体
struct Book {
long id;
char name[MAX_SIZE];
float price;
};
// 初始化图书表
void init(struct Book bookTable[]) {
// 初始化5本书
bookTable[0].id = 1;
strcpy(bookTable[0].name, "book1");
bookTable[0].price = 78;
bookTable[1].id = 2;
strcpy(bookTable[1].name, "book2");
bookTable[1].price = 54;
bookTable[2].id = 3;
strcpy(bookTable[2].name, "book3");
bookTable[2].price = 45;
bookTable[3].id = 4;
strcpy(bookTable[3].name, "book4");
bookTable[3].price = 98;
bookTable[4].id = 5;
strcpy(bookTable[4].name, "book5");
bookTable[4].price = 76;
}
// 插入书
void insert(struct Book bookTable[], int index, struct Book book) {
// 移动后面的书
for (int i = 4; i >= index; i--) {
bookTable[i + 1] = bookTable[i];
}
// 插入新书
bookTable[index] = book;
}
// 删除书
void delete(struct Book bookTable[], int index) {
// 移动后面的书
for (int i = index; i < 4; i++) {
bookTable[i] = bookTable[i + 1];
}
}
// 获取书
struct Book get(struct Book bookTable[], int index) {
return bookTable[index];
}
// 获取最贵的书
struct Book getMax(struct Book bookTable[]) {
struct Book max = bookTable[0];
for (int i = 1; i < 5; i++) {
if (bookTable[i].price > max.price) {
max = bookTable[i];
}
}
return max;
}
// 获取最便宜的书
struct Book getMin(struct Book bookTable[]) {
struct Book min = bookTable[0];
for (int i = 1; i < 5; i++) {
if (bookTable[i].price < min.price) {
min = bookTable[i];
}
}
return min;
}
int main() {
struct Book bookTable[10];
init(bookTable);
struct Book book1 = {11, "python程序设计", 23};
struct Book book2 = {12, "数据结构", 55};
insert(bookTable, 4, book1); // 在5的位置上插入python程序设计
insert(bookTable, 10, book2); // 在11的位置上插入数据结构
delete(bookTable, 1); // 删除第2本书
struct Book book3 = get(bookTable, 2); // 获取第3本书
printf("第3本书是:%ld %s %.0f\n", book3.id, book3.name, book3.price);
struct Book maxBook = getMax(bookTable);
printf("最大值:%s\n", maxBook.name);
struct Book minBook = getMin(bookTable);
printf("最小值:%s\n", minBook.name);
// 打印图书信息
for (int i = 0; i < 10; i++) {
if (bookTable[i].id != 0) {
printf("%ld %s %.0f\n", bookTable[i].id, bookTable[i].name, bookTable[i].price);
}
}
return 0;
}
```
程序执行结果:
```
第3本书是:3 book3 45
最大值:book4
最小值:book10
1 book1 78
3 book3 45
4 book4 98
11 python程序设计 23
5 book5 76
6 book6 12
7 book7 14
8 book8 17
9 book9 58
12 数据结构 55
```
相关推荐
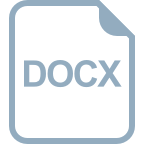
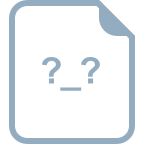
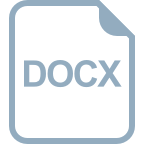
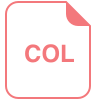
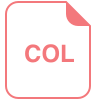













