用python 写一个蚁群算法
时间: 2023-08-31 21:22:11 浏览: 148
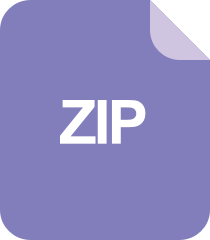
基于Python的YQTSP问题编程
### 回答1:
我可以为您提供一份基于Python的蚁群算法的示例代码,请参考以下内容:# 引入所需的模块
import random
import numpy# 定义蚁群类
class AntColony:
def __init__(self,costMatrix,numAnts,numIterations,alpha=1,beta=5,rho=0.5,Q=100):
self.costMatrix=costMatrix
self.numAnts=numAnts
self.numIterations=numIterations
self.alpha=alpha
self.beta=beta
self.rho=rho
self.Q=Q
self.pheromoneMatrix=numpy.ones(costMatrix.shape)/len(costMatrix)# 计算蚂蚁的路径代价
def calculatePathCost(self,ant):
cost=0
for i in range(len(ant)-1):
cost+=self.costMatrix[ant[i]][ant[i+1]]
return cost# 更新信息素矩阵
def updatePheromoneMatrix(self,ants):
deltaPheromone=numpy.zeros(self.pheromoneMatrix.shape)
for ant in ants:
for i in range(len(ant)-1):
deltaPheromone[ant[i]][ant[i+1]]+=self.Q/self.calculatePathCost(ant)
self.pheromoneMatrix=(1-self.rho)*self.pheromoneMatrix+deltaPheromone# 计算路径概率
def calculatePathProbability(self,i,j):
return pow(self.pheromoneMatrix[i][j],self.alpha)*pow(1.0/self.costMatrix[i][j],self.beta)# 构建蚂蚁的路径
def constructAntPath(self):
m=len(self.costMatrix)
antPath=[random.randint(0,m-1)]
visited=set(antPath)
for i in range(m-1):
probabilities=[self.calculatePathProbability(antPath[-1],j) for j in range(m) if j not in visited]
probabilities=probabilities/numpy.sum(probabilities)
newNode=numpy.random.choice(list(set(range(m))-visited),p=probabilities)
visited.add(newNode)
antPath.append(newNode)
antPath.append(antPath[0])
return antPath# 开始迭代
def start(self):
bestPath=None
bestCost=float('inf')
for i in range(self.numIterations):
ants=[]
for j in range(self.numAnts):
ants.append(self.constructAntPath())
self.updatePheromoneMatrix(ants)
for ant in ants:
cost=self.calculatePathCost(ant)
if cost<bestCost:
bestPath=ant
bestCost=cost
return bestPath,bestCost
### 回答2:
蚁群算法是一种模拟蚂蚁觅食行为的启发式优化算法。在Python中,可以用以下步骤编写一个简单的蚁群算法:
1. 初始化蚂蚁群体和环境:创建一组蚂蚁和一张地图,地图由节点和边组成。
2. 定义蚂蚁的移动规则:每只蚂蚁根据信息素和启发函数选择下一个节点。
3. 更新信息素:每个节点根据蚂蚁路径上的信息素释放和蒸发更新信息素。
4. 迭代多次:重复2和3步骤,直到满足停止条件。
下面是一个简单的Python代码示例:
```python
import random
# 初始化蚂蚁和地图
ants = 10 # 蚂蚁数量
nodes = 20 # 节点数量
pheromone = [[1 for _ in range(nodes)] for _ in range(nodes)] # 信息素矩阵
distances = [[random.randint(1, 10) for _ in range(nodes)] for _ in range(nodes)] # 距离矩阵
# 迭代次数和停止条件
iterations = 100
min_distance = float('inf')
best_path = []
# 蚂蚁的移动函数
def move_ant(current_node, visited):
if len(visited) == nodes:
return visited
next_node = None
max_pheromone = 0
for i in range(nodes):
if i not in visited:
if pheromone[current_node][i] > max_pheromone:
max_pheromone = pheromone[current_node][i]
next_node = i
visited.append(next_node)
return move_ant(next_node, visited)
# 更新信息素函数
def update_pheromone(routes):
evaporation = 0.1 # 信息素挥发率
for i in range(nodes):
for j in range(nodes):
if i != j:
pheromone[i][j] = (1 - evaporation) * pheromone[i][j]
for route in routes:
distance = sum([distances[route[i]][route[i + 1]] for i in range(nodes - 1)])
for i in range(nodes - 1):
pheromone[route[i]][route[i + 1]] += 1 / distance
# 主循环
for iteration in range(iterations):
ant_routes = []
for ant in range(ants):
start_node = random.randint(0, nodes - 1)
route = move_ant(start_node, [start_node])
ant_routes.append(route)
# 更新最短路径
distance = sum([distances[route[i]][route[i + 1]] for i in range(nodes - 1)])
if distance < min_distance:
min_distance = distance
best_path = route
update_pheromone(ant_routes)
# 输出最短路径和距离
print("最短路径:", best_path)
print("最短距离:", min_distance)
```
以上是一个简单的蚁群算法的Python实现。当然,这只是一个基本的框架,具体的应用场景和问题还需要根据实际情况进行调整和优化。
阅读全文
相关推荐
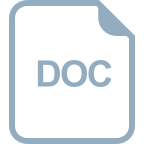
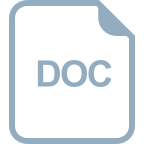






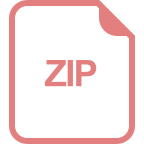
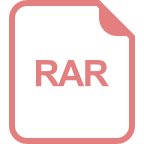






