用python写一段蚁群算法实例
时间: 2023-10-01 13:08:39 浏览: 103
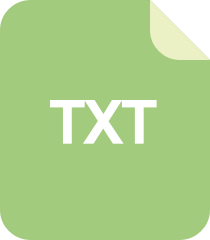
蚁群算法小例
好的,以下是用python实现基本的蚁群算法的示例代码:
```python
import random
# 蚂蚁类
class Ant:
def __init__(self, start, end, graph):
self.current_node = start
self.end_node = end
self.graph = graph
self.path = [start]
self.distance = 0.0
# 蚂蚁走一步
def move(self, alpha, beta, pheromone):
next_node = self.select_next(alpha, beta, pheromone)
self.path.append(next_node)
self.distance += self.graph[self.current_node][next_node]
self.current_node = next_node
# 选择下一个节点
def select_next(self, alpha, beta, pheromone):
# 如果当前节点是终点,直接返回
if self.current_node == self.end_node:
return self.end_node
next_node = None
# 计算每个节点的概率
probabilities = {}
denominator = 0.0
for node in range(len(self.graph)):
if node not in self.path:
numerator = (pheromone[self.current_node][node] ** alpha) * \
((1.0 / self.graph[self.current_node][node]) ** beta)
probabilities[node] = numerator
denominator += numerator
# 根据概率选择下一个节点
if denominator == 0.0:
next_node = random.choice(list(set(range(len(self.graph))) - set(self.path)))
else:
probabilities = {node: prob / denominator for node, prob in probabilities.items()}
probability_sum = 0.0
target_probability = random.random()
for node, prob in probabilities.items():
probability_sum += prob
if probability_sum >= target_probability:
next_node = node
break
return next_node
# 蚁群算法函数
def ant_colony_algorithm(graph, start, end, ant_count=10, alpha=1.0, beta=2.0, evaporation=0.5, pheromone_deposit=1.0,
max_iterations=50):
best_distance = float('inf')
best_path = None
pheromone = [[1.0 for _ in range(len(graph))] for _ in range(len(graph))]
# 循环迭代
for iteration in range(max_iterations):
# 初始化蚂蚁
ants = [Ant(start, end, graph) for _ in range(ant_count)]
# 蚁群行动
for ant in ants:
while ant.current_node != ant.end_node:
ant.move(alpha, beta, pheromone)
if ant.distance < best_distance:
best_distance = ant.distance
best_path = ant.path
# 更新信息素
for i, row in enumerate(pheromone):
for j, col in enumerate(row):
pheromone[i][j] *= (1.0 - evaporation)
for ant in ants:
if j == ant.path[i + 1]:
pheromone[i][j] += (pheromone_deposit / ant.distance)
return best_path, best_distance
# 示例
graph = [[0, 3, 4, 5], [3, 0, 2, 3], [4, 2, 0, 1], [5, 3, 1, 0]]
start = 0
end = 3
path, distance = ant_colony_algorithm(graph, start, end)
print('最短路径: ', path)
print('最短距离: ', distance)
```
该示例代码实现了一个对一个简单的四节点图求解最短路径的蚁群算法。在实际应用时需要针对具体问题进行适当的参数调整和算法优化。
阅读全文
相关推荐
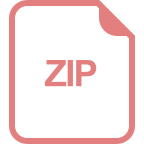
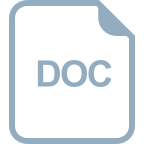
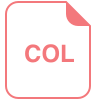
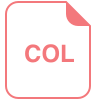
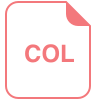
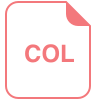
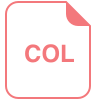
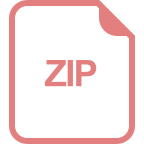
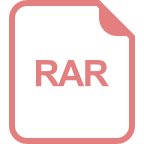
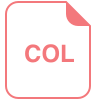
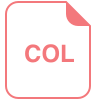
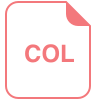
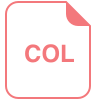
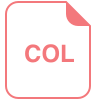
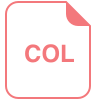
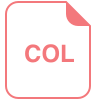