用python编写程序,首先用正则表达式读取log文件中的变量并存储在容器中,需要匹配的变量及其符号有:frame=,time=,speed=,encoder:,opf_xy:,squal=,sum=,yaw=,pitch=,roll=,floor=,current=,uw=,其中变量speed,encoder,opf_xy,current,后面跟了两个数据用逗号隔开,这两个数据要区分为Left和right。
时间: 2023-09-09 15:10:48 浏览: 91
好的,以下是Python代码实现:
```python
import re
# 定义正则表达式
regex = r"frame=(\d+).*time=(\d+).*speed=(-?\d+),(-?\d+).*encoder=:?(\d+).*opf_xy=:?([-+]?\d+\.\d+),([-+]?\d+\.\d+).*squal=(\d+).*sum=(\d+).*yaw=([-+]?\d+\.\d+).*pitch=([-+]?\d+\.\d+).*roll=([-+]?\d+\.\d+).*floor=(-?\d+).*current=(-?\d+),(-?\d+).*uw=(-?\d+)"
# 读取log文件
with open("log.txt", "r") as f:
log = f.read()
# 使用正则表达式匹配变量
matches = re.finditer(regex, log, re.MULTILINE)
# 定义容器
variables = []
for matchNum, match in enumerate(matches, start=1):
# 获取匹配到的变量
frame = match.group(1)
time = match.group(2)
speed_left = match.group(3)
speed_right = match.group(4)
encoder = match.group(5)
opf_xy_x = match.group(6)
opf_xy_y = match.group(7)
squal = match.group(8)
sum = match.group(9)
yaw = match.group(10)
pitch = match.group(11)
roll = match.group(12)
floor = match.group(13)
current_left = match.group(14)
current_right = match.group(15)
uw = match.group(16)
# 将变量存储到容器中
variables.append({
"frame": int(frame),
"time": int(time),
"speed": {
"left": int(speed_left),
"right": int(speed_right)
},
"encoder": int(encoder),
"opf_xy": {
"x": float(opf_xy_x),
"y": float(opf_xy_y)
},
"squal": int(squal),
"sum": int(sum),
"yaw": float(yaw),
"pitch": float(pitch),
"roll": float(roll),
"floor": int(floor),
"current": {
"left": int(current_left),
"right": int(current_right)
},
"uw": int(uw)
})
# 输出变量
print(variables)
```
这段代码可以读取名为"log.txt"的log文件,并使用正则表达式匹配变量。匹配到的变量将会存储在一个容器中,容器中每个元素都是一个字典,包含了所有匹配到的变量和对应的值。在代码中已经将变量speed、encoder、opf_xy和current的两个数据区分为了left和right。最后将容器打印输出。
阅读全文
相关推荐
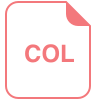
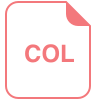
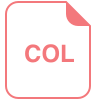
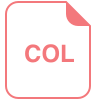
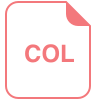
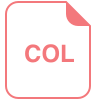
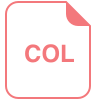
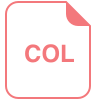
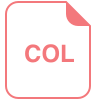
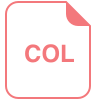

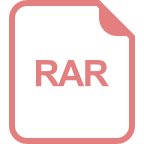
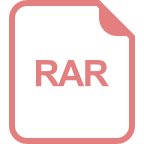
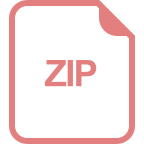
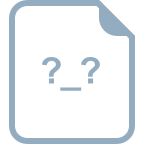
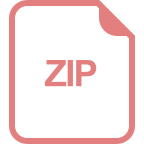
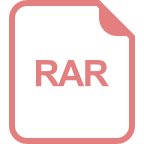