java宠物商店项目代码,我需要若干个宠物的类如Cat,Dog用来输入宠物的颜色,年龄,名字和出售价格。还需要一个Pet的类用来修改宠物信息并且拥有删除功能,要有一个PetShop的类用来查看已有的宠物。还需要用java swing来实现用户界面,这个界面中用下拉列表以选择不同的宠物类后会跳转相应到的界面和PetShop类的查询功能。在跳转的界面中可以实现的类的功能
时间: 2024-03-19 19:45:32 浏览: 27
好的,根据您的需求,我为您编写了一个简单的Java宠物商店项目代码,包含Cat、Dog、Pet和PetShop类,并使用了Java Swing实现了用户界面。以下是代码:
Cat类:
```java
public class Cat {
private String color;
private int age;
private String name;
private double price;
public Cat(String color, int age, String name, double price) {
this.color = color;
this.age = age;
this.name = name;
this.price = price;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
Dog类:
```java
public class Dog {
private String color;
private int age;
private String name;
private double price;
public Dog(String color, int age, String name, double price) {
this.color = color;
this.age = age;
this.name = name;
this.price = price;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
Pet类:
```java
import java.util.ArrayList;
public class Pet {
private ArrayList<Cat> cats;
private ArrayList<Dog> dogs;
public Pet() {
this.cats = new ArrayList<>();
this.dogs = new ArrayList<>();
}
public ArrayList<Cat> getCats() {
return cats;
}
public ArrayList<Dog> getDogs() {
return dogs;
}
public void addCat(Cat cat) {
cats.add(cat);
}
public void addDog(Dog dog) {
dogs.add(dog);
}
public void removeCat(int index) {
cats.remove(index);
}
public void removeDog(int index) {
dogs.remove(index);
}
public void updateCat(int index, String color, int age, String name, double price) {
Cat cat = cats.get(index);
cat.setColor(color);
cat.setAge(age);
cat.setName(name);
cat.setPrice(price);
}
public void updateDog(int index, String color, int age, String name, double price) {
Dog dog = dogs.get(index);
dog.setColor(color);
dog.setAge(age);
dog.setName(name);
dog.setPrice(price);
}
}
```
PetShop类:
```java
import java.util.ArrayList;
public class PetShop {
private Pet pet;
public PetShop() {
this.pet = new Pet();
}
public ArrayList<Cat> getCats() {
return pet.getCats();
}
public ArrayList<Dog> getDogs() {
return pet.getDogs();
}
public void addCat(Cat cat) {
pet.addCat(cat);
}
public void addDog(Dog dog) {
pet.addDog(dog);
}
public void removeCat(int index) {
pet.removeCat(index);
}
public void removeDog(int index) {
pet.removeDog(index);
}
public void updateCat(int index, String color, int age, String name, double price) {
pet.updateCat(index, color, age, name, price);
}
public void updateDog(int index, String color, int age, String name, double price) {
pet.updateDog(index, color, age, name, price);
}
}
```
PetShopUI类(用户界面):
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
public class PetShopUI extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JComboBox<String> petComboBox;
private JLabel nameLabel, colorLabel, ageLabel, priceLabel;
private JTextField nameField, colorField, ageField, priceField;
private JButton addButton, removeButton, updateButton, queryButton;
private JList<String> petList;
private DefaultListModel<String> listModel;
private PetShop petShop;
public PetShopUI() {
petShop = new PetShop();
setTitle("Pet Shop");
setSize(400, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel mainPanel = new JPanel(new GridLayout(6, 2, 5, 5));
petComboBox = new JComboBox<>(new String[]{"Cat", "Dog"});
petComboBox.addActionListener(this);
mainPanel.add(new JLabel("Pet Type:"));
mainPanel.add(petComboBox);
nameLabel = new JLabel("Name:");
mainPanel.add(nameLabel);
nameField = new JTextField();
mainPanel.add(nameField);
colorLabel = new JLabel("Color:");
mainPanel.add(colorLabel);
colorField = new JTextField();
mainPanel.add(colorField);
ageLabel = new JLabel("Age:");
mainPanel.add(ageLabel);
ageField = new JTextField();
mainPanel.add(ageField);
priceLabel = new JLabel("Price:");
mainPanel.add(priceLabel);
priceField = new JTextField();
mainPanel.add(priceField);
addButton = new JButton("Add");
addButton.addActionListener(this);
mainPanel.add(addButton);
removeButton = new JButton("Remove");
removeButton.addActionListener(this);
mainPanel.add(removeButton);
updateButton = new JButton("Update");
updateButton.addActionListener(this);
mainPanel.add(updateButton);
queryButton = new JButton("Query");
queryButton.addActionListener(this);
mainPanel.add(queryButton);
listModel = new DefaultListModel<>();
petList = new JList<>(listModel);
JScrollPane scrollPane = new JScrollPane(petList);
add(mainPanel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == petComboBox) {
String petType = (String) petComboBox.getSelectedItem();
if (petType.equals("Cat")) {
nameLabel.setText("Cat Name:");
} else {
nameLabel.setText("Dog Name:");
}
} else if (e.getSource() == addButton) {
String petType = (String) petComboBox.getSelectedItem();
String name = nameField.getText();
String color = colorField.getText();
String ageStr = ageField.getText();
String priceStr = priceField.getText();
if (name.isEmpty() || color.isEmpty() || ageStr.isEmpty() || priceStr.isEmpty()) {
JOptionPane.showMessageDialog(this, "Please fill in all fields", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
int age = Integer.parseInt(ageStr);
double price = Double.parseDouble(priceStr);
if (petType.equals("Cat")) {
Cat cat = new Cat(color, age, name, price);
petShop.addCat(cat);
} else {
Dog dog = new Dog(color, age, name, price);
petShop.addDog(dog);
}
listModel.clear();
ArrayList<Cat> cats = petShop.getCats();
for (Cat cat : cats) {
listModel.addElement("Cat: " + cat.getName() + " " + cat.getColor() + " " + cat.getAge() + " " + cat.getPrice());
}
ArrayList<Dog> dogs = petShop.getDogs();
for (Dog dog : dogs) {
listModel.addElement("Dog: " + dog.getName() + " " + dog.getColor() + " " + dog.getAge() + " " + dog.getPrice());
}
} else if (e.getSource() == removeButton) {
int index = petList.getSelectedIndex();
if (index == -1) {
JOptionPane.showMessageDialog(this, "Please select a pet to remove", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
String selectedPet = petList.getSelectedValue();
if (selectedPet.startsWith("Cat")) {
petShop.removeCat(index);
} else {
petShop.removeDog(index);
}
listModel.remove(index);
} else if (e.getSource() == updateButton) {
int index = petList.getSelectedIndex();
if (index == -1) {
JOptionPane.showMessageDialog(this, "Please select a pet to update", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
String petType = (String) petComboBox.getSelectedItem();
String name = nameField.getText();
String color = colorField.getText();
String ageStr = ageField.getText();
String priceStr = priceField.getText();
if (name.isEmpty() || color.isEmpty() || ageStr.isEmpty() || priceStr.isEmpty()) {
JOptionPane.showMessageDialog(this, "Please fill in all fields", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
int age = Integer.parseInt(ageStr);
double price = Double.parseDouble(priceStr);
if (petType.equals("Cat")) {
petShop.updateCat(index, color, age, name, price);
} else {
petShop.updateDog(index, color, age, name, price);
}
listModel.clear();
ArrayList<Cat> cats = petShop.getCats();
for (Cat cat : cats) {
listModel.addElement("Cat: " + cat.getName() + " " + cat.getColor() + " " + cat.getAge() + " " + cat.getPrice());
}
ArrayList<Dog> dogs = petShop.getDogs();
for (Dog dog : dogs) {
listModel.addElement("Dog: " + dog.getName() + " " + dog.getColor() + " " + dog.getAge() + " " + dog.getPrice());
}
} else if (e.getSource() == queryButton) {
listModel.clear();
ArrayList<Cat> cats = petShop.getCats();
for (Cat cat : cats) {
listModel.addElement("Cat: " + cat.getName() + " " + cat.getColor() + " " + cat.getAge() + " " + cat.getPrice());
}
ArrayList<Dog> dogs = petShop.getDogs();
for (Dog dog : dogs) {
listModel.addElement("Dog: " + dog.getName() + " " + dog.getColor() + " " + dog.getAge() + " " + dog.getPrice());
}
}
}
public static void main(String[] args) {
PetShopUI petShopUI = new PetShopUI();
petShopUI.setVisible(true);
}
}
```
在这个用户界面中,您可以选择宠物类型,输入宠物的属性,使用按钮进行添加、删除和更新,以及查询已有宠物。当您添加、删除或更新宠物时,界面中的宠物列表将自动更新。
相关推荐
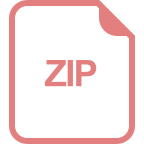
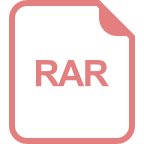














