ch32v307vct6配置串口
时间: 2023-08-30 09:05:23 浏览: 167
对于 ch32v307vct6 芯片来说,配置串口需要以下步骤:
1. 确定串口配置参数,包括波特率、数据位、校验位和停止位等。
2. 配置 GPIO,将对应的引脚设置为串口功能。
3. 配置串口控制器,包括设置波特率、数据位、校验位和停止位等参数,以及开启串口发送和接收中断。
4. 编写相应的串口发送和接收函数,用于向串口发送数据和接收数据。
下面是一个简单的示例代码,演示如何配置 ch32v307vct6 的串口:
```c
#include "ch32v307.h"
#define UART_TX_PIN GPIO_Pin_10
#define UART_RX_PIN GPIO_Pin_11
void uart_init(uint32_t baud_rate, uint8_t data_bits, uint8_t parity, uint8_t stop_bits)
{
// 使能 GPIO 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置 GPIO 引脚为复用功能
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = UART_TX_PIN | UART_RX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 使能 USART 时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
// 配置 USART 参数
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = baud_rate;
USART_InitStructure.USART_WordLength = data_bits;
USART_InitStructure.USART_Parity = parity;
USART_InitStructure.USART_StopBits = stop_bits;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
// 开启 USART 中断
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
// 使能 USART
USART_Cmd(USART2, ENABLE);
}
void uart_send_byte(uint8_t byte)
{
while (USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
USART_SendData(USART2, byte);
}
uint8_t uart_receive_byte(void)
{
while (USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART2);
}
```
在上面的代码中,我们首先定义了 UART_TX_PIN 和 UART_RX_PIN 分别表示串口发送和接收引脚所在的 GPIO 引脚号。
然后,在 uart_init 函数中,我们依次进行了 GPIO 配置、USART 配置和中断配置等操作。其中,baud_rate、data_bits、parity 和 stop_bits 分别表示波特率、数据位、校验位和停止位等参数。
最后,我们分别定义了 uart_send_byte 和 uart_receive_byte 两个函数,用于向串口发送数据和接收数据。
阅读全文
相关推荐
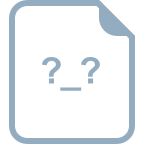




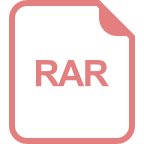
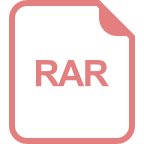
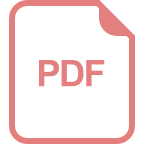
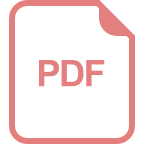
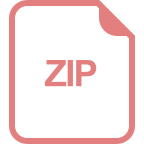
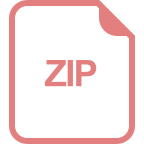
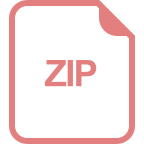
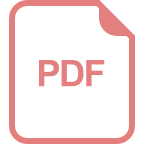
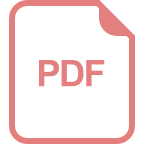
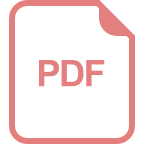
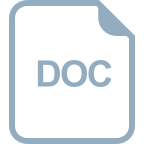
