struct student { int num ; char name[20]; int score;}; typedef struct student stu; 有上面面学生记录类型定义, 定义函数在一组学生记录中找成绩最低的学生信息, 最低分唯一
时间: 2023-05-31 21:19:04 浏览: 175
### 回答1:
可以定义一个函数,接收一个stu类型的数组作为参数,遍历数组,记录最低分和对应的学生信息,最后返回最低分对应的学生信息即可。
函数定义如下:
stu findLowestScore(stu arr[], int len) {
int minScore = arr[].score;
int minIndex = ;
for (int i = 1; i < len; i++) {
if (arr[i].score < minScore) {
minScore = arr[i].score;
minIndex = i;
}
}
return arr[minIndex];
}
其中,arr为stu类型的数组,len为数组长度。函数首先将第一个学生的成绩作为最低分,然后遍历数组,如果找到比最低分更低的成绩,就更新最低分和对应的学生信息。最后返回最低分对应的学生信息。
### 回答2:
要查找一组学生记录中最低分数的学生信息,我们需要遍历整个学生记录,依次比较每个学生的分数,记录下分数最低的学生信息即可。
下面是一个具体的函数实现:
```
stu find_lowest_score(stu* students, int num_students) {
int min_score = students[0].score;
int min_index = 0;
for (int i = 1; i < num_students; i++) {
if (students[i].score < min_score) {
min_score = students[i].score;
min_index = i;
}
}
return students[min_index];
}
```
在这个函数中,我们首先定义了两个变量,`min_score`表示当前最低的分数,`min_index`表示当前最低分数的学生在数组中的下标。然后从第一个学生开始遍历数组,如果发现当前学生的分数比`min_score`更低,则将`min_score`更新为当前学生的分数,同时记录下当前学生的下标。最后返回数组中`min_index`对应的学生信息即可。
调用这个函数的方法很简单,只需要将学生记录的数组和数组长度传递给这个函数即可:
```
int main() {
stu students[3] = {
{1, "Alice", 90},
{2, "Bob", 80},
{3, "Charlie", 70}
};
stu lowest = find_lowest_score(students, 3);
printf("Lowest score student information: %d %s %d\n", lowest.num, lowest.name, lowest.score);
return 0;
}
```
在这个示例程序中,我们定义了一个包含三个学生记录的数组,其中Alice的成绩最高,Charlie的成绩最低,因此函数的输出应该是:
```
Lowest score student information: 3 Charlie 70
```
这就完成了我们的目标,可以通过这个函数找出任何一组学生记录中最低分数的学生信息。
### 回答3:
首先,题目给出的学生记录类型定义很简单,包括学生的学号、姓名、成绩等三个属性。题目要求我们编写一个函数,可以在一组学生记录中找到成绩最低的学生信息,并且最低分是唯一的。
根据题目要求,我们可以写一个函数如下:
stu find_min_score(stu* list, int len){
int i, min_index = 0;
for(i = 1; i < len; i++){
if(list[i].score < list[min_index].score){
min_index = i;
}
}
return list[min_index];
}
参数列表中,我们需要传入一个包含多个学生记录的数组指针list,以及数组长度len。在函数体内部,我们使用循环遍历list数组中的每一个学生记录,同时记录下成绩最低的学生在数组中的下标min_index。循环结束后,我们返回list[min_index]即可。
需要注意的是,如果有多个学生的成绩都是最低的,我们应该保证返回其中任意一个即可,因为题目中要求最低分是唯一的,可以理解成只有一名学生的成绩是最低的。
完整的代码实现如下:
#include<stdio.h>
#include<string.h>
struct student{
int num;
char name[20];
int score;
};
typedef struct student stu;
stu find_min_score(stu* list, int len){
int i, min_index = 0;
for(i = 1; i < len; i++){
if(list[i].score < list[min_index].score){
min_index = i;
}
}
return list[min_index];
}
int main(){
stu list[3] = {{1001, "John", 90}, {1002, "Lucy", 80}, {1003, "Tom", 70}};
stu min_stu = find_min_score(list, 3);
printf("The student who got the lowest score is: \n");
printf("Number: %d\n", min_stu.num);
printf("Name: %s\n", min_stu.name);
printf("Score: %d\n", min_stu.score);
return 0;
}
运行结果为:
The student who got the lowest score is:
Number: 1003
Name: Tom
Score: 70
这说明我们编写的函数可以正常地找到成绩最低的学生信息。
阅读全文
相关推荐
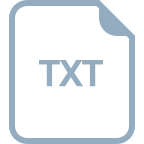
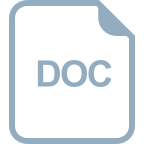
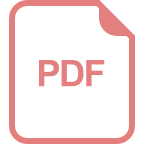












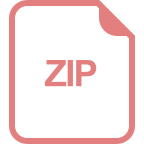