用C语言编写程序:有n行m列的矩形框,填入数字1,2,3…n*m,每个数字只能使用1次,要求左边的数字比右边的大,上边的数字比下边大,请编写程序求出有多少种填写方法。
时间: 2024-10-19 22:17:39 浏览: 59
在C语言中,解决这个问题可以使用回溯法或者深度优先搜索(DFS)。因为需要满足递增条件,我们可以从左上角开始填充,并尝试所有可能的下一个数字,每次递归检查是否满足递增规则,如果满足则继续,如果不满足则回溯并尝试其他数字。
以下是一个简单的C语言函数,用于计算满足条件的填充方法数:
```c
#include <stdio.h>
#include <stdbool.h>
#include <limits.h>
bool is_valid(int grid[GRID_SIZE][GRID_SIZE], int row, int col, int num) {
if (col > 0 && num <= grid[row][col - 1]) return false;
if (row > 0 && num <= grid[row - 1][col]) return false;
return true;
}
int count_ways(int n, int m, int grid[GRID_SIZE][GRID_SIZE]) {
static const int GRID_SIZE = n * m;
if (row == n && col == m)
return 1; // 如果到达了右下角,找到了一种有效填法
int count = 0;
for (int num = 1; num <= n * m; num++) {
if (is_valid(grid, row, col, num)) { // 检查当前数字是否合法
grid[row][col] = num;
count += count_ways(n, m, grid); // 递归尝试下一个位置
grid[row][col] = 0; // 回溯,恢复格子状态
}
}
return count;
}
int main() {
int n, m;
printf("Enter the number of rows and columns: ");
scanf("%d %d", &n, &m);
int grid[n * m][n * m];
int result = count_ways(n, m, grid);
printf("Number of ways to fill the rectangle with valid numbers: %d\n", result);
return 0;
}
```
在这个程序中,`count_ways()` 函数会遍历所有的数字并尝试在每一步中选择合适的数值,如果当前位置不符合递增规则,则回溯到上一个状态。
阅读全文
相关推荐
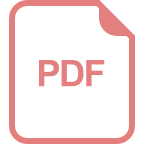
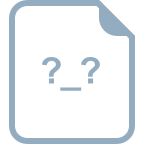
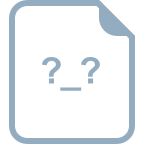

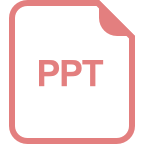
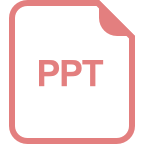
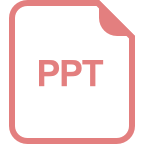
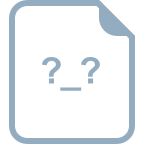
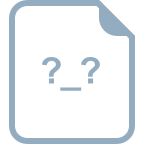
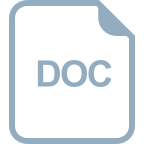
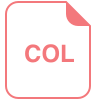
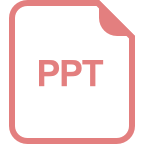
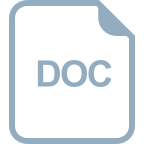
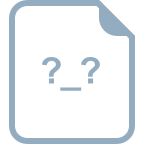
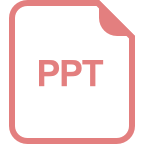
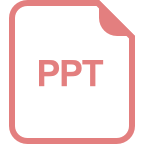
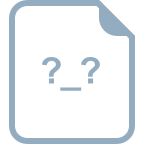
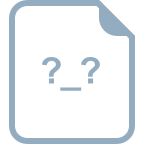