pythonopencv,将1.jpg和2.jpg拼接/融合成一张图片,并保存为output.jpg 1. 偏移量通过特征点匹配自动计算 2. 拼接后空白区域用黑色代替
时间: 2024-03-13 20:43:51 浏览: 34
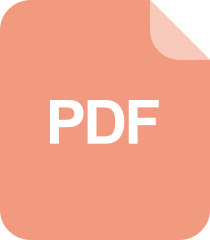
python opencv进行图像拼接
好的,我可以帮你解决这个问题。你需要先安装OpenCV库,然后使用以下代码:
```python
import cv2
import numpy as np
# 读取图片
img1 = cv2.imread('1.jpg')
img2 = cv2.imread('2.jpg')
# 创建SIFT对象
sift = cv2.xfeatures2d.SIFT_create()
# 检测关键点和描述符
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 创建FLANN匹配器对象
matcher = cv2.FlannBasedMatcher()
# 匹配关键点
matches = matcher.match(des1, des2)
# 根据匹配结果筛选出较好的匹配点
matches = sorted(matches, key=lambda x: x.distance)
good_matches = matches[:10]
# 获取匹配点的坐标
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 计算变换矩阵
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 计算图像1在图像2中的偏移量
h, w = img1.shape[:2]
pts = np.float32([[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]).reshape(-1, 1, 2)
dst = cv2.perspectiveTransform(pts, M)
offset_x, offset_y = np.int32(dst.min(axis=0).ravel() - 0.5)
# 计算拼接后的图像大小
dst_w = img2.shape[1] + offset_x
dst_h = max(img2.shape[0], img1.shape[0] + offset_y)
# 创建拼接后的图像
dst = np.zeros((dst_h, dst_w, 3), dtype=np.uint8)
dst[:img2.shape[0], :img2.shape[1]] = img2
# 将图像1拼接到图像2中
M[0, 2] += offset_x
M[1, 2] += offset_y
img1_warp = cv2.warpPerspective(img1, M, (dst_w, dst_h))
dst[offset_y:offset_y + h, offset_x:offset_x + w] = img1_warp
# 保存拼接后的图像
cv2.imwrite('output.jpg', dst)
```
这段代码中,我们使用SIFT算法检测图像中的关键点和描述符,并使用FLANN匹配器进行匹配。然后根据匹配点计算图像1和图像2之间的变换矩阵,进而计算出图像1在图像2中的偏移量。最后创建一个新的图像,将图像2和图像1拼接到一起,并保存为output.jpg。在拼接时,我们使用黑色填充空白区域。
阅读全文
相关推荐
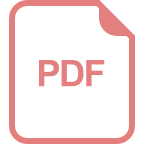


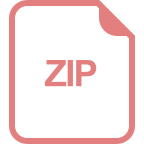

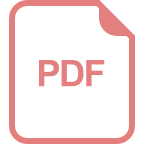
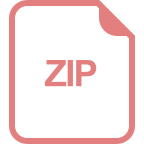
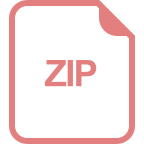
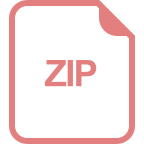
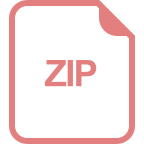