用PYTHON怎么读取OBJ文件
时间: 2023-07-08 08:04:40 浏览: 331
使用 Python 读取 OBJ 文件可以使用以下步骤:
1. 打开 OBJ 文件:
```python
with open('model.obj', 'r') as f:
lines = f.readlines()
```
2. 遍历读取每一行的数据
```python
vertices = []
faces = []
for line in lines:
if line.startswith('v '):
vertex = list(map(float, line.split()[1:]))
vertices.append(vertex)
elif line.startswith('f '):
face = [int(x.split('/')[0]) for x in line.split()[1:]]
faces.append(face)
```
上述代码中,我们使用了 `startswith()` 方法来判断每一行的类型,根据 `v ` 和 `f ` 来分别读取顶点和面的数据。
3. 将顶点和面的数据存储在 Numpy 数组中
```python
import numpy as np
vertices = np.array(vertices)
faces = np.array(faces) - 1 # OBJ 文件中面的索引从 1 开始,需要减去 1
```
最终,我们将顶点和面的数据存储在 Numpy 数组中,方便后续的处理和使用。
完整代码如下:
```python
import numpy as np
with open('model.obj', 'r') as f:
lines = f.readlines()
vertices = []
faces = []
for line in lines:
if line.startswith('v '):
vertex = list(map(float, line.split()[1:]))
vertices.append(vertex)
elif line.startswith('f '):
face = [int(x.split('/')[0]) for x in line.split()[1:]]
faces.append(face)
vertices = np.array(vertices)
faces = np.array(faces) - 1
```
阅读全文
相关推荐

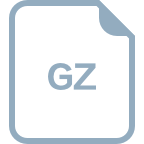















