实验内容:接口、多态 1、编写2个接口:InterfaceA和InterfaceB;在接口InterfaceA中有个方法void printCapitalLetter();在接口InterfaceB中有个方法void printLowercaseLetter();然后写一个类Print实现接口InterfaceA和InterfaceB,要求printCapitalLetter()方法 实现输出大写英文字母表的功能,printLowercaseLetter()方法实现输出小写英文字母表的功能。再写一个主类E,在主类E的main方法中创建Print的对象并赋值给InterfaceA的变量a,对象a调用printCapitalLetter方法;最后再在主类E的main方法中创建Print的对象并赋值给InterfaceB的变量b,对象b调用printLowercaseLetter方法。 2、按要求编写Java程序: (1)编写一个接口:InterfaceA,只含有一个方法int method(int n); (2)编写一个类:ClassA来实现接口InterfaceA,实现int method(int n)接口方法时,要求计算1到n的和; (3)编写另一个类:ClassB来实现接口InterfaceA,实现int method(int n)接口方法时,要求计算n的阶乘(n!); (4)编写测试类E,在测试类E的main方法中使用接口回调的形式来测试实现接口的类。
时间: 2024-03-02 20:52:54 浏览: 79
1. 编写两个接口 InterfaceA 和 InterfaceB:
```
public interface InterfaceA {
void printCapitalLetter();
}
public interface InterfaceB {
void printLowercaseLetter();
}
```
2. 编写一个实现接口 InterfaceA 和 InterfaceB 的类 Print:
```
public class Print implements InterfaceA, InterfaceB {
@Override
public void printCapitalLetter() {
for (char c = 'A'; c <= 'Z'; c++) {
System.out.print(c + " ");
}
System.out.println();
}
@Override
public void printLowercaseLetter() {
for (char c = 'a'; c <= 'z'; c++) {
System.out.print(c + " ");
}
System.out.println();
}
}
```
3. 在主类 E 中使用 Print 的对象分别赋值给 InterfaceA 和 InterfaceB 的变量,并调用相应的方法:
```
public class E {
public static void main(String[] args) {
InterfaceA a = new Print();
a.printCapitalLetter();
InterfaceB b = new Print();
b.printLowercaseLetter();
}
}
```
4. 编写一个接口 InterfaceA 和两个实现类 ClassA 和 ClassB,分别计算 1 到 n 的和和 n 的阶乘:
```
public interface InterfaceA {
int method(int n);
}
public class ClassA implements InterfaceA {
@Override
public int method(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
}
public class ClassB implements InterfaceA {
@Override
public int method(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
}
```
5. 在测试类 E 的 main 方法中使用接口回调的形式来测试实现接口的类:
```
public class E {
public static void main(String[] args) {
InterfaceA a = new ClassA();
System.out.println("1+2+...+n 的和为:" + a.method(10));
InterfaceA b = new ClassB();
System.out.println("n 的阶乘为:" + b.method(5));
}
}
```
阅读全文
相关推荐
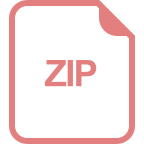
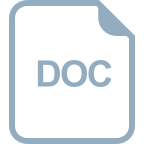
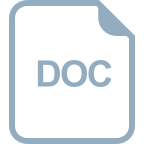
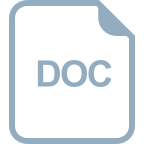
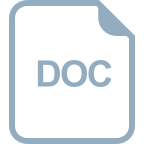
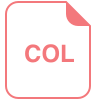
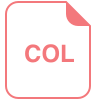
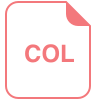
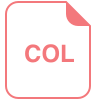
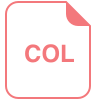
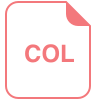
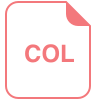



