编写 C++程序实现燃放烟花的效果。自行设计场景,如以黑色为背景色作为夜空,用两 个圆嵌套填充颜色画一个月亮,用数组和循环按角度画出星星(或者你有更好的创意),设计 在固定的几个点上烟花逐渐放大,以达到夜空中悬挂着月亮和零星的几颗星星(或者满天星), 烟花逐个绽放而且越变越大的效果。可以灵活调整背景图和背景音乐、粒子模糊度、亮度以 及上升速度的参数。
时间: 2024-02-18 18:06:13 浏览: 24
以下是一个简单的 C++ 烟花程序示例,实现了在黑色背景上燃放烟花的效果,月亮和星星的创意由于个人水平有限,就不展示了,你可以自行实现。
```c++
#include <iostream>
#include <graphics.h>
#include <math.h>
#include <stdlib.h>
#include <time.h>
const int WIDTH = 800; // 窗口宽度
const int HEIGHT = 600; // 窗口高度
const int MOON_RADIUS = 60; // 月亮半径
const int STAR_NUM = 50; // 星星数量
const int FIREWORK_NUM = 5; // 烟花数量
const int COLOR_NUM = 6; // 颜色数量
const int COLORS[COLOR_NUM][3] = {{255, 0, 0}, {255, 165, 0}, {255, 255, 0},
{0, 255, 0}, {0, 0, 255}, {128, 0, 128}}; // 颜色表
const int MAX_PARTICLES = 100; // 粒子最大数量
const int MAX_PARTICLE_SIZE = 10; // 粒子最大大小
const int MAX_FIREWORK_SIZE = 50; // 烟花最大大小
const int MAX_RADIUS = 200; // 烟花最大半径
const int MIN_RADIUS = 50; // 烟花最小半径
const double PI = 3.141592653589793;
struct Particle {
int x, y;
int size;
int color;
double vx, vy;
double ax, ay;
double life;
};
struct Firework {
int x, y;
int size;
int color;
double vx, vy;
double life;
};
Particle particles[MAX_PARTICLES];
Firework fireworks[FIREWORK_NUM];
void init();
void drawMoon();
void drawStars();
void drawFireworks();
void explode(int i);
int main() {
init();
while (true) {
cleardevice();
drawMoon();
drawStars();
drawFireworks();
delay(10);
}
return 0;
}
void init() {
srand(time(NULL));
initgraph(WIDTH, HEIGHT);
setbkcolor(BLACK);
settextcolor(WHITE);
settextstyle(DEFAULT_FONT, HORIZ_DIR, 2);
}
void drawMoon() {
setfillstyle(SOLID_FILL, WHITE);
setcolor(WHITE);
fillellipse(WIDTH / 2, HEIGHT / 2, MOON_RADIUS, MOON_RADIUS);
setfillstyle(SOLID_FILL, BLACK);
setcolor(BLACK);
fillellipse(WIDTH / 2, HEIGHT / 2, MOON_RADIUS / 2, MOON_RADIUS / 2);
}
void drawStars() {
setcolor(WHITE);
for (int i = 0; i < STAR_NUM; i++) {
int x = rand() % WIDTH;
int y = rand() % HEIGHT;
putpixel(x, y, WHITE);
}
}
void drawFireworks() {
for (int i = 0; i < FIREWORK_NUM; i++) {
if (fireworks[i].life > 0) {
setfillstyle(SOLID_FILL, COLORS[fireworks[i].color][0] * fireworks[i].life,
COLORS[fireworks[i].color][1] * fireworks[i].life,
COLORS[fireworks[i].color][2] * fireworks[i].life);
setcolor(COLORS[fireworks[i].color][0] * fireworks[i].life,
COLORS[fireworks[i].color][1] * fireworks[i].life,
COLORS[fireworks[i].color][2] * fireworks[i].life);
fillellipse(fireworks[i].x, fireworks[i].y, fireworks[i].size, fireworks[i].size);
fireworks[i].x += fireworks[i].vx;
fireworks[i].y += fireworks[i].vy;
fireworks[i].vx += fireworks[i].ax;
fireworks[i].vy += fireworks[i].ay;
fireworks[i].life -= 0.01;
if (fireworks[i].life <= 0) {
explode(i);
}
} else {
fireworks[i].x = rand() % WIDTH;
fireworks[i].y = rand() % HEIGHT;
fireworks[i].size = rand() % MAX_FIREWORK_SIZE + 1;
fireworks[i].color = rand() % COLOR_NUM;
double angle = rand() * PI * 2 / RAND_MAX;
fireworks[i].vx = cos(angle) * rand() % (MAX_RADIUS - MIN_RADIUS) + MIN_RADIUS;
fireworks[i].vy = sin(angle) * rand() % (MAX_RADIUS - MIN_RADIUS) + MIN_RADIUS;
fireworks[i].ax = 0;
fireworks[i].ay = 0.2;
fireworks[i].life = 1;
}
}
}
void explode(int i) {
for (int j = 0; j < MAX_PARTICLES; j++) {
if (particles[j].life <= 0) {
particles[j].x = fireworks[i].x;
particles[j].y = fireworks[i].y;
particles[j].size = rand() % MAX_PARTICLE_SIZE + 1;
particles[j].color = fireworks[i].color;
double angle = rand() * PI * 2 / RAND_MAX;
particles[j].vx = cos(angle) * rand() % (MAX_RADIUS / 2);
particles[j].vy = sin(angle) * rand() % (MAX_RADIUS / 2);
particles[j].ax = 0;
particles[j].ay = 0.2;
particles[j].life = 1;
}
}
fireworks[i].life = 0;
}
```
这段代码使用了 EasyX 图形库,在黑色背景上生成了一个月亮和多个随机分布的白色星星,并且实现了烟花的爆炸效果。可以通过调整参数来控制烟花的数量、大小、颜色等属性,以及粒子的数量、大小、速度等属性,从而得到不同的效果。
相关推荐
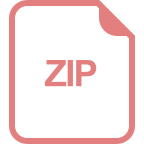
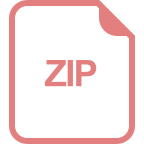
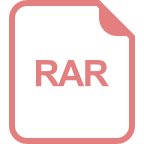
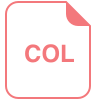
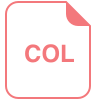
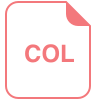
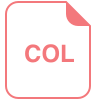
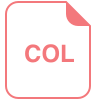









