python小游戏大球吃小球
时间: 2023-07-14 08:02:21 浏览: 152
好的,我会为你编写一个简单的Python小游戏,名为"大球吃小球"。在这个游戏中,你将控制一个大球,不断地吃掉随机生成的小球来增加得分。当大球吃掉小球时,得分增加,小球会重新生成在随机位置上。游戏结束的条件是大球碰到边界或者自己的身体。
下面是游戏的代码实现:
```python
import pygame
import sys
import random
# 初始化游戏
pygame.init()
# 定义游戏窗口大小和背景颜色
window_width = 800
window_height = 600
bg_color = (255, 255, 255)
# 定义大球的初始位置、大小和移动速度
ball_pos = [window_width/2, window_height/2]
ball_radius = 20
ball_color = (255, 0, 0)
ball_speed = 5
# 定义小球的初始位置、大小和移动速度
small_ball_pos = [random.randint(0, window_width), random.randint(0, window_height)]
small_ball_radius = 10
small_ball_color = (0, 0, 255)
small_ball_speed = 3
# 定义得分
score = 0
# 创建游戏窗口
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("大球吃小球")
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 移动大球
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
ball_pos[0] -= ball_speed
if keys[pygame.K_RIGHT]:
ball_pos[0] += ball_speed
if keys[pygame.K_UP]:
ball_pos[1] -= ball_speed
if keys[pygame.K_DOWN]:
ball_pos[1] += ball_speed
# 判断大球是否碰到边界
if ball_pos[0] - ball_radius < 0 or ball_pos[0] + ball_radius > window_width or \
ball_pos[1] - ball_radius < 0 or ball_pos[1] + ball_radius > window_height:
print("游戏结束,得分:", score)
pygame.quit()
sys.exit()
# 判断大球是否吃到小球
distance = ((ball_pos[0] - small_ball_pos[0]) ** 2 + (ball_pos[1] - small_ball_pos[1]) ** 2) ** 0.5
if distance <= ball_radius + small_ball_radius:
score += 1
small_ball_pos = [random.randint(0, window_width), random.randint(0, window_height)]
# 绘制游戏界面
window.fill(bg_color)
pygame.draw.circle(window, ball_color, (int(ball_pos[0]), int(ball_pos[1])), ball_radius)
pygame.draw.circle(window, small_ball_color, (int(small_ball_pos[0]), int(small_ball_pos[1])), small_ball_radius)
pygame.display.update()
```
你可以将上述代码保存为一个.py文件,并运行它来开始游戏。在游戏中,你可以使用键盘的方向键来控制大球的移动。当大球吃到小球时,得分会增加。当大球碰到边界或者自己的身体时,游戏结束并显示得分。
希望这个小游戏能满足你的需求!如果有任何问题,请随时告诉我。
相关推荐
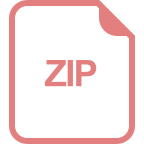














